java vue项目,请求网址: http://localhost:9876/api/User。还是前端网址,我怎么调成后端,具体方法
时间: 2023-08-07 07:38:57 浏览: 59
如果你的项目是前后端分离的,那么你需要先确保后端服务已经启动并监听在9876端口上。如果后端服务已经启动,你可以在前端代码中找到发送请求的地方,将请求的地址修改为后端服务的地址即可。
具体来说,如果你使用的是axios库进行网络请求,可以在axios的配置中将baseURL设置为后端服务的地址,然后在发送请求时只需要填写相对路径即可。示例代码如下:
```
import axios from 'axios'
const instance = axios.create({
baseURL: 'http://localhost:9876/api'
})
// 发送请求
instance.get('/User')
.then(response => {
console.log(response.data)
})
.catch(error => {
console.log(error)
})
```
这样就可以将前端请求发送到后端服务,获取后端返回的数据了。
相关问题
前端采用vue,后端采用springboot+微服务+网关,进行对接时出现以下报错怎么解决Access to XMLHttpRequest at 'http://127.0.0.1:8090/user/admin/register' from origin 'http://localhost:8080' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource.
在Vue前端和Spring Boot后端中解决CORS问题,你可以尝试以下方法:
1. 后端配置CORS过滤器:在Spring Boot后端的网关服务中,添加一个CORS过滤器,处理跨域请求。你可以创建一个类实现`javax.servlet.Filter`接口,然后在`doFilter()`方法中设置响应头,包括`Access-Control-Allow-Origin`字段。示例代码如下:
```java
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@Component
public class CorsFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) request;
HttpServletResponse httpResponse = (HttpServletResponse) response;
httpResponse.setHeader("Access-Control-Allow-Origin", "*");
httpResponse.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
httpResponse.setHeader("Access-Control-Max-Age", "3600");
httpResponse.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization, X-Requested-With");
if ("OPTIONS".equalsIgnoreCase(httpRequest.getMethod())) {
httpResponse.setStatus(HttpServletResponse.SC_OK);
} else {
chain.doFilter(request, response);
}
}
}
```
2. 配置Spring Security:如果你在后端使用了Spring Security来进行身份验证和授权,你可以在配置类中添加以下代码来解决CORS问题:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.cors().and().csrf().disable();
}
@Bean
public CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(Arrays.asList("Content-Type", "Authorization", "X-Requested-With"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
```
这样配置后,Spring Security将允许来自任何源的跨域请求。
3. 使用代理:在开发环境下,你可以在Vue的配置文件中设置代理,将前端请求转发到后端服务,从而避免浏览器的CORS限制。在`vue.config.js`文件中添加以下代码:
```javascript
module.exports = {
devServer: {
proxy: {
'/api': {
target: 'http://127.0.0.1:8090', // 后端服务地址
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
}
}
```
然后,在前端代码中使用`/api`作为请求的基础路径,例如:`axios.post('/api/user/admin/register', data)`。
这些方法中的任何一种都可以帮助你解决CORS问题。你可以根据你的具体情况选择适合的方法。
SpringBoot+vue前端后端如何在登录后请求头上加上token,请给我写出详细具体的步骤以及代码
好的,下面是 Spring Boot 后端和 Vue.js 前端的示例代码和步骤。
1. 在 Spring Boot 后端,我们可以使用 Spring Security 框架来实现基于 token 的认证和授权。
首先,在 pom.xml 文件中添加 Spring Security 的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
其次,我们需要创建一个类来实现 Spring Security 的配置,例如:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private JwtAuthenticationEntryPoint unauthorizedHandler;
@Bean
public JwtAuthenticationFilter authenticationTokenFilterBean() throws Exception {
return new JwtAuthenticationFilter();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.cors().and().csrf().disable()
.exceptionHandling().authenticationEntryPoint(unauthorizedHandler).and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS).and()
.authorizeRequests()
.antMatchers("/api/auth/**").permitAll()
.anyRequest().authenticated();
// 添加 JWT token 过滤器
http.addFilterBefore(authenticationTokenFilterBean(), UsernamePasswordAuthenticationFilter.class);
}
}
```
在上面的代码中,我们配置了 Spring Security 的一些基本参数,例如:使用 BCryptPasswordEncoder 进行密码加密,实现 UserDetailsService 接口来加载用户信息,配置 JWT token 过滤器等。
2. 在用户登录成功后,生成一个 token 并返回给前端。
在 Spring Boot 后端,我们可以使用 JWT token 来实现认证和授权。在用户登录成功后,我们需要生成一个 JWT token 并返回给前端,例如:
```java
// 生成 JWT token
String token = Jwts.builder()
.setSubject(user.getUsername())
.setExpiration(new Date(System.currentTimeMillis() + EXPIRATION_TIME))
.signWith(SignatureAlgorithm.HS512, SECRET)
.compact();
// 将 token 返回给前端
response.addHeader(HEADER_STRING, TOKEN_PREFIX + token);
```
在上面的代码中,我们使用 Jwts 类创建一个 JWT token,设置 token 的过期时间和签名算法,并将 token 添加到响应头中返回给前端。其中,HEADER_STRING 和 TOKEN_PREFIX 是我们自定义的常量。
3. 在前端,我们需要在请求头中添加 Authorization 字段,值为 "Bearer " + token。
在 Vue.js 前端,我们可以使用 axios 库来发送 HTTP 请求,并在请求头中添加 Authorization 字段。
首先,我们需要在 main.js 文件中设置 axios 的默认配置,例如:
```javascript
import axios from 'axios'
axios.defaults.baseURL = 'http://localhost:8080/api'
axios.defaults.headers.common['Content-Type'] = 'application/json'
// 在请求头中添加 Authorization 字段
axios.interceptors.request.use(
config => {
const token = localStorage.getItem('token')
if (token) {
config.headers.common['Authorization'] = 'Bearer ' + token
}
return config
},
error => {
return Promise.reject(error)
}
)
```
在上面的代码中,我们设置了 axios 的默认请求 URL 和请求头的 Content-Type。并在 axios 的请求拦截器中,判断本地存储中是否有 token,如果有,则将 token 添加到请求头的 Authorization 字段中。
4. 在后端,我们需要从请求头中解析出 token,然后使用 JWT token 进行验证和授权。
在 Spring Boot 后端,我们需要从请求头中解析出 Authorization 字段,然后使用 JWT token 进行验证和授权。
例如,在 JwtAuthenticationFilter 类中,我们可以重写 doFilterInternal 方法,从请求头中解析出 token,并将 token 传递给 JwtTokenUtil 类进行验证和授权,例如:
```java
@Component
public class JwtAuthenticationFilter extends OncePerRequestFilter {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws ServletException, IOException {
String header = request.getHeader(HEADER_STRING);
String username = null;
String authToken = null;
if (header != null && header.startsWith(TOKEN_PREFIX)) {
authToken = header.replace(TOKEN_PREFIX, "");
try {
username = jwtTokenUtil.getUsernameFromToken(authToken);
} catch (IllegalArgumentException e) {
logger.error("an error occurred during getting username from token", e);
} catch (ExpiredJwtException e) {
logger.warn("the token is expired and not valid anymore", e);
}
} else {
logger.warn("couldn't find bearer string, will ignore the header");
}
if (username != null && SecurityContextHolder.getContext().getAuthentication() == null) {
UserDetails userDetails = userDetailsService.loadUserByUsername(username);
if (jwtTokenUtil.validateToken(authToken, userDetails)) {
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
authentication.setDetails(new WebAuthenticationDetailsSource().buildDetails(request));
logger.info("authenticated user " + username + ", setting security context");
SecurityContextHolder.getContext().setAuthentication(authentication);
}
}
chain.doFilter(request, response);
}
}
```
在上面的代码中,我们首先从请求头中解析出 Authorization 字段,然后使用 JwtTokenUtil 类的 getUsernameFromToken 方法解析出 token 中的用户名。接着,我们使用 UserDetailsService 接口加载用户信息,并使用 JwtTokenUtil 类的 validateToken 方法进行 token 的验证和授权。最后,我们将用户信息设置到 Spring Security 的上下文中,以便后续的请求可以进行访问控制。
至此,我们就完成了 Spring Boot 后端和 Vue.js 前端如何在登录后请求头上加上 token 的示例代码和步骤。
相关推荐
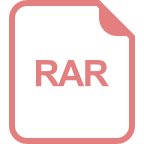
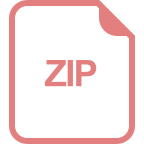
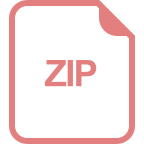












