java
时间: 2023-10-11 21:23:06 浏览: 31
好的,那么你可以按照以下步骤来实现统计关键字在一篇文章中出现的次数的功能:
1. 读取指定的文本文件。
2. 从控制台获取用户输入的关键字。
3. 统计关键字在文本文件中出现的次数。
4. 将统计结果追加到原文本文件后面。
下面是一个示例代码:
```java
import java.io.*;
public class KeywordCounter {
public static void main(String[] args) {
// 1. 读取指定的文本文件
File file = new File("wenzhang.txt");
StringBuilder sb = new StringBuilder();
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append(System.lineSeparator());
}
} catch (IOException e) {
e.printStackTrace();
}
String content = sb.toString();
// 2. 从控制台获取用户输入的关键字
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("请输入关键字:");
String keyword = "";
try {
keyword = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
// 3. 统计关键字在文本文件中出现的次数
int count = 0;
int index = 0;
while (true) {
index = content.indexOf(keyword, index);
if (index != -1) {
count++;
index += keyword.length();
} else {
break;
}
}
// 4. 将统计结果追加到原文本文件后面
try (BufferedWriter bw = new BufferedWriter(new FileWriter(file, true))) {
bw.write("关键字 \"" + keyword + "\" 出现了 " + count + " 次。");
bw.newLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("关键字 \"" + keyword + "\" 出现了 " + count + " 次。");
}
}
```
注意,以上代码仅供参考,你需要根据自己的实际情况进行适当的修改。另外,为了方便起见,我将统计结果直接输出到了控制台和文本文件中,你可以根据需要进行调整。
相关推荐
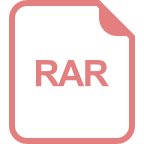
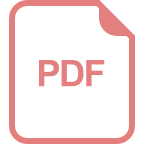
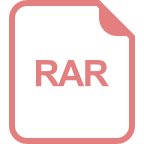















