统计指定子字符串在字符中出现的次数
时间: 2023-11-28 19:04:21 浏览: 48
好的,可以使用 Python 编程语言来实现这个功能。可以使用字符串的 `count()` 方法来计算指定子字符串在目标字符串中出现的次数。例如,假设目标字符串为 `s`,指定子字符串为 `sub_str`,则可以使用以下代码来实现统计功能:
```python
s = "hello world, hello python, hello AI"
sub_str = "hello"
count = s.count(sub_str)
print(count) # 输出 3
```
上述代码中,首先定义了目标字符串 `s` 和指定子字符串 `sub_str`,然后使用 `count()` 方法统计 `sub_str` 在 `s` 中出现的次数,并将结果保存在变量 `count` 中。最后将结果输出即可。
需要注意的是,`count()` 方法是区分大小写的,如果需要忽略大小写可以先将目标字符串和子字符串都转换为小写或大写形式,再进行统计。
相关问题
统计指定子字符串在字符中出现的次数Java实现
可以使用Java中的String类的indexOf()和substring()方法来实现统计指定子字符串在字符串中出现的次数。具体实现如下:
```java
public static int countSubstring(String str, String subStr) {
int count = 0;
int index = 0;
while ((index = str.indexOf(subStr, index)) != -1) {
count++;
index += subStr.length();
}
return count;
}
```
该方法接收两个参数:原始字符串和要统计的子字符串。它使用一个while循环来遍历整个字符串,每次使用indexOf()方法查找子字符串出现的位置,如果找到了就将计数器加1,然后将查找的起始位置更新为当前位置加上子字符串长度,以便查找下一个子字符串。
示例用法:
```java
String str = "hello world, world is beautiful!";
String subStr = "world";
System.out.println(countSubstring(str, subStr)); // 输出2
```
注意:该方法不区分大小写,如果需要区分大小写可以使用String的equalsIgnoreCase()方法和substring()方法。
c++统计字符串出现的次数
可以使用 std::string 类来存储字符串,然后使用 std::count 函数来统计字符串出现的次数。
下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
int main()
{
std::string str = "hello world, world";
std::string substr = "world";
// 统计子字符串出现的次数
int count = std::count(str.begin(), str.end(), substr.begin(), substr.end());
std::cout << "The substring \"" << substr << "\" appears " << count << " times in the string \"" << str << "\"." << std::endl;
return 0;
}
```
输出结果为:
```
The substring "world" appears 2 times in the string "hello world, world".
```
其中,std::count 函数的前两个参数是指定要查找的字符串的范围,第三个和第四个参数是指定要查找的子字符串的范围。
相关推荐
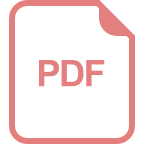
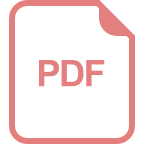
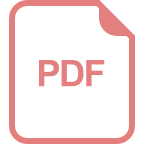












