用Java生成sm2密钥
时间: 2023-04-03 15:01:02 浏览: 121
可以使用Bouncy Castle库来生成SM2密钥对,具体实现可以参考以下代码:
import org.bouncycastle.crypto.AsymmetricCipherKeyPair;
import org.bouncycastle.crypto.generators.ECKeyPairGenerator;
import org.bouncycastle.crypto.params.ECKeyGenerationParameters;
import org.bouncycastle.crypto.params.ECPrivateKeyParameters;
import org.bouncycastle.crypto.params.ECPublicKeyParameters;
import org.bouncycastle.crypto.util.PrivateKeyFactory;
import org.bouncycastle.crypto.util.PublicKeyFactory;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.math.ec.ECPoint;
import java.security.Security;
public class SM2KeyGenerator {
static {
Security.addProvider(new BouncyCastleProvider());
}
public static void main(String[] args) throws Exception {
// 生成SM2密钥对
AsymmetricCipherKeyPair keyPair = generateSM2KeyPair();
// 获取SM2公钥
ECPublicKeyParameters publicKey = (ECPublicKeyParameters) keyPair.getPublic();
ECPoint q = publicKey.getQ();
byte[] publicKeyBytes = q.getEncoded(false);
// 获取SM2私钥
ECPrivateKeyParameters privateKey = (ECPrivateKeyParameters) keyPair.getPrivate();
byte[] privateKeyBytes = privateKey.getD().toByteArray();
System.out.println("SM2公钥:" + bytesToHex(publicKeyBytes));
System.out.println("SM2私钥:" + bytesToHex(privateKeyBytes));
}
/**
* 生成SM2密钥对
*/
public static AsymmetricCipherKeyPair generateSM2KeyPair() {
ECKeyPairGenerator keyPairGenerator = new ECKeyPairGenerator();
ECKeyGenerationParameters keyGenerationParams = new ECKeyGenerationParameters(SM2Util.DOMAIN_PARAMS, SM2Util.getRandom());
keyPairGenerator.init(keyGenerationParams);
return keyPairGenerator.generateKeyPair();
}
/**
* 字节数组转十六进制字符串
*/
public static String bytesToHex(byte[] bytes) {
StringBuilder hex = new StringBuilder();
for (byte b : bytes) {
hex.append(String.format("%02x", b));
}
return hex.toString();
}
}
相关推荐
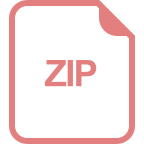
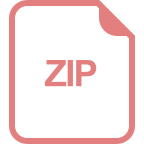














