javaspringboot制作微信支付,中使用apiv3和apiv2的区别
时间: 2024-05-27 18:11:04 浏览: 375
在JavaSpringBoot中使用微信支付时,可以选择使用APIv3或APIv2版本,它们之间的区别如下:
1. APIv3是微信支付的最新版本,采用了更加安全和高效的签名算法和证书管理方式,支持RSA和HMAC-SHA256两种签名算法,可以使用证书和密钥进行签名和验签,保证了支付过程的安全性。
2. APIv2是微信支付的旧版本,采用了MD5签名算法和API密钥进行签名和验签,存在安全性问题,容易被攻击。同时,APIv2的接口文档较为简单,使用起来相对容易。
3. 在使用APIv3版本时,需要先申请微信支付商户平台的APIv3密钥和证书,以及设置支付回调通知地址等参数,相对来说比较繁琐。而APIv2版本则不需要申请证书和密钥,只需要在商户平台设置API密钥即可。
综上所述,如果考虑支付过程的安全性和高效性,推荐使用APIv3版本;如果对安全性要求不高,或者对技术要求不高,可以使用APIv2版本。
相关问题
javaspringboot创建微信支付,使用apiv2 JSAPI支付代码怎么写
下面是一个简单的Java Spring Boot微信支付示例,使用APIv2 JSAPI支付代码:
1. 创建微信支付配置类
```java
@Configuration
public class WechatPayConfig {
@Value("${wechat.pay.appId}")
private String appId;
@Value("${wechat.pay.mchId}")
private String mchId;
@Value("${wechat.pay.apiKey}")
private String apiKey;
@Bean
public WXPay wxPay() throws Exception {
WXPayConfigImpl config = WXPayConfigImpl.getInstance();
config.setAppID(appId);
config.setMchID(mchId);
config.setKey(apiKey.getBytes());
return new WXPay(config);
}
}
```
2. 创建微信支付控制器
```java
@RestController
@RequestMapping("/api/wechat/pay")
public class WechatPayController {
@Autowired
private WXPay wxPay;
@PostMapping("/unifiedorder")
public ResponseEntity<?> unifiedOrder(@RequestBody WechatPayRequest request, HttpServletRequest httpRequest) {
try {
// 构造支付请求参数
Map<String, String> data = new HashMap<>();
data.put("body", request.getBody());
data.put("out_trade_no", request.getOutTradeNo());
data.put("total_fee", request.getTotalFee().toString());
data.put("spbill_create_ip", httpRequest.getRemoteAddr());
data.put("notify_url", "https://your-notify-url.com");
data.put("trade_type", "JSAPI");
data.put("openid", request.getOpenid());
// 调用统一下单API
Map<String, String> result = wxPay.unifiedOrder(data);
// 生成JSAPI支付参数
String prepayId = result.get("prepay_id");
Map<String, String> jsApiParams = new HashMap<>();
jsApiParams.put("appId", wxPay.getConfig().getAppID());
jsApiParams.put("timeStamp", String.valueOf(System.currentTimeMillis() / 1000));
jsApiParams.put("nonceStr", WXPayUtil.generateNonceStr());
jsApiParams.put("package", "prepay_id=" + prepayId);
jsApiParams.put("signType", "MD5");
String paySign = WXPayUtil.generateSignature(jsApiParams, wxPay.getConfig().getKey());
jsApiParams.put("paySign", paySign);
return ResponseEntity.ok(jsApiParams);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.getMessage());
}
}
}
```
3. 创建微信支付请求类
```java
public class WechatPayRequest {
private String body;
private String outTradeNo;
private BigDecimal totalFee;
private String openid;
// getters and setters
}
```
4. 在application.properties文件中添加微信支付配置
```properties
wechat.pay.appId=your-appId
wechat.pay.mchId=your-mchId
wechat.pay.apiKey=your-apiKey
```
5. 使用Postman等工具测试JSAPI支付接口
发送POST请求到http://localhost:8080/api/wechat/pay/unifiedorder,请求体如下:
```json
{
"body": "测试支付",
"outTradeNo": "20220101000001",
"totalFee": 1,
"openid": "your-openid"
}
```
其中,openid是JSAPI支付必须的参数,可以通过微信OAuth2.0授权获取。
返回结果如下:
```json
{
"appId": "your-appId",
"timeStamp": "1641260647",
"nonceStr": "9jvOvEJZzVw2STN5",
"package": "prepay_id=wx01155047389241f9c9ab15d8d8e9813000",
"signType": "MD5",
"paySign": "B24F9D7F2DBE3048F7BA4D400C4B0B4B"
}
```
将返回结果中的数据传递给微信JSAPI支付接口即可完成支付。
java springboot构建微信支付,使用apiv2密钥jsapi支付方式代码怎么写
以下是使用apiv2密钥jsapi支付方式的Java Spring Boot代码示例:
1. 创建微信支付配置类
```java
@Configuration
public class WxPayConfig {
@Value("${wxpay.appid}")
private String appId;
@Value("${wxpay.mchid}")
private String mchId;
@Value("${wxpay.key}")
private String key;
@Value("${wxpay.notifyurl}")
private String notifyUrl;
@Bean
public WxPayService wxPayService() {
WxPayService wxPayService = new WxPayServiceImpl();
WxPayConfig payConfig = new WxPayConfig();
payConfig.setAppId(appId);
payConfig.setMchId(mchId);
payConfig.setMchKey(key);
payConfig.setNotifyUrl(notifyUrl);
wxPayService.setConfig(payConfig);
return wxPayService;
}
}
```
2. 编写Controller接口
```java
@RestController
@RequestMapping("/wxpay")
public class WxPayController {
@Autowired
private WxPayService wxPayService;
@PostMapping("/jsapi")
public ResultCode<Map<String, String>> jsapiPay(@RequestBody WxPayDto wxPayDto) throws WxPayException {
WxPayUnifiedOrderRequest request = new WxPayUnifiedOrderRequest();
request.setBody(wxPayDto.getBody());
request.setOutTradeNo(wxPayDto.getOutTradeNo());
request.setTotalFee(wxPayDto.getTotalFee());
request.setSpbillCreateIp(wxPayDto.getSpbillCreateIp());
request.setNotifyUrl(wxPayService.getConfig().getNotifyUrl());
request.setTradeType(WxPayConstants.TradeType.JSAPI);
request.setOpenid(wxPayDto.getOpenid());
WxPayUnifiedOrderResult result = wxPayService.unifiedOrder(request);
Map<String, String> jsApiParams = wxPayService.getJsapiPayParams(result.getPrepayId());
return ResultCode.success(jsApiParams);
}
}
```
3. 编写WxPayDto类(用于接收支付请求参数)
```java
@Data
public class WxPayDto {
@NotEmpty(message = "商品描述不能为空")
private String body;
@NotEmpty(message = "商户订单号不能为空")
private String outTradeNo;
@NotNull(message = "订单金额不能为空")
private Integer totalFee;
@NotEmpty(message = "终端IP不能为空")
private String spbillCreateIp;
@NotEmpty(message = "openid不能为空")
private String openid;
}
```
4. 在application.yml文件中添加微信支付配置
```yaml
wxpay:
appid: your_appid # 微信公众号appid
mchid: your_mchid # 商户号
key: your_key # 商户密钥
notifyurl: your_notify_url # 支付成功回调地址
```
以上代码示例仅供参考,具体实现方式可能会因业务需求而异。
阅读全文
相关推荐
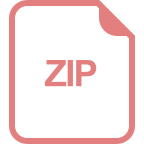
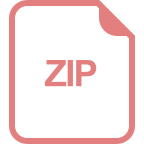
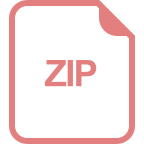

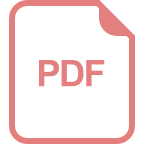
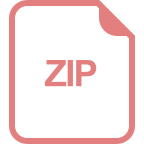
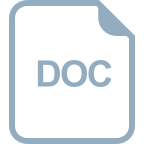
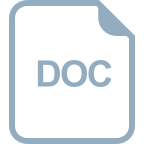








