vistual studio io 形式 ffmpeg 保存ts文件
时间: 2023-07-21 12:36:27 浏览: 46
在 Visual Studio 中使用 FFmpeg 保存 TS 文件,您需要将 FFmpeg 库添加到您的项目中,并使用 C++ 代码编写文件保存功能。
以下是一个简单的示例程序,它使用 FFmpeg 库将输入文件保存为 MPEG-TS 文件:
```cpp
extern "C"
{
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
}
int save_as_ts(const char* input_file, const char* output_file)
{
AVFormatContext* input_format_ctx = NULL;
AVFormatContext* output_format_ctx = NULL;
AVPacket pkt;
int ret;
av_register_all();
// Open input file
if ((ret = avformat_open_input(&input_format_ctx, input_file, NULL, NULL)) < 0) {
return ret;
}
// Retrieve stream information
if ((ret = avformat_find_stream_info(input_format_ctx, NULL)) < 0) {
return ret;
}
// Open output file
if ((ret = avformat_alloc_output_context2(&output_format_ctx, NULL, NULL, output_file)) < 0) {
return ret;
}
// Open output file for writing
if ((ret = avio_open(&output_format_ctx->pb, output_file, AVIO_FLAG_WRITE)) < 0) {
return ret;
}
// Write stream headers
if ((ret = avformat_write_header(output_format_ctx, NULL)) < 0) {
return ret;
}
// Loop through input packets
while (av_read_frame(input_format_ctx, &pkt) >= 0) {
// Write packet to output file
if ((ret = av_interleaved_write_frame(output_format_ctx, &pkt)) < 0) {
return ret;
}
// Free packet
av_packet_unref(&pkt);
}
// Write stream trailer
av_write_trailer(output_format_ctx);
// Close input file
avformat_close_input(&input_format_ctx);
// Close output file
avio_close(output_format_ctx->pb);
avformat_free_context(output_format_ctx);
return 0;
}
```
此代码使用 FFmpeg 库的 AVFormatContext 结构体打开输入文件和输出文件,并使用 av_read_frame() 和 av_interleaved_write_frame() 函数循环读取输入文件中的数据包,并将其写入输出文件。请注意,此代码假定输入文件和输出文件都具有单个音频/视频流。
您可以在 Visual Studio 中创建一个 C++ 项目,并将 FFmpeg 库和头文件添加到项目中。然后,您可以将此代码添加到您的项目中,并调用 save_as_ts() 函数来保存输入文件。
相关推荐
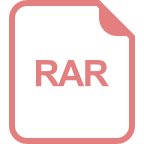













