编写一个Java程序,应用接口计算图形的面积、周长、表面积、体积。建立一个接口,再建长方形、圆、长方体类实现接口,完成面积、周长、表面积、体积的计算,并输出结果。
时间: 2023-08-26 21:03:57 浏览: 95
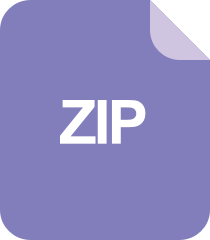
wtz.zip_java小程序_圆的面积
下面是一个Java程序,实现了接口计算图形的面积、周长、表面积、体积。
Shape.java(接口文件):
```
public interface Shape {
double getArea(); // 计算面积
double getPerimeter(); // 计算周长
}
public interface SolidShape {
double getSurfaceArea(); // 计算表面积
double getVolume(); // 计算体积
}
```
Rectangle.java(长方形类):
```
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double getArea() {
return length * width;
}
@Override
public double getPerimeter() {
return 2 * (length + width);
}
}
```
Circle.java(圆类):
```
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
```
Cuboid.java(长方体类):
```
public class Cuboid implements Shape, SolidShape {
private double length;
private double width;
private double height;
public Cuboid(double length, double width, double height) {
this.length = length;
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return 2 * (length * width + length * height + width * height);
}
@Override
public double getPerimeter() {
return 4 * (length + width + height);
}
@Override
public double getSurfaceArea() {
return 2 * (length * width + length * height + width * height);
}
@Override
public double getVolume() {
return length * width * height;
}
}
```
Main.java(主程序文件):
```
public class Main {
public static void main(String[] args) {
Shape rectangle = new Rectangle(5, 10);
System.out.println("长方形面积:" + rectangle.getArea());
System.out.println("长方形周长:" + rectangle.getPerimeter());
Shape circle = new Circle(5);
System.out.println("圆面积:" + circle.getArea());
System.out.println("圆周长:" + circle.getPerimeter());
SolidShape cuboid = new Cuboid(5, 10, 15);
System.out.println("长方体面积:" + cuboid.getSurfaceArea());
System.out.println("长方体体积:" + cuboid.getVolume());
}
}
```
输出结果:
```
长方形面积:50.0
长方形周长:30.0
圆面积:78.53981633974483
圆周长:31.41592653589793
长方体面积:850.0
长方体体积:750.0
```
阅读全文
相关推荐
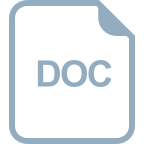
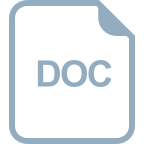
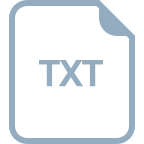
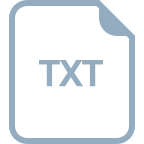
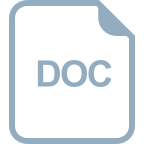
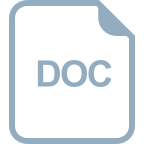
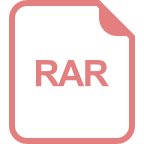
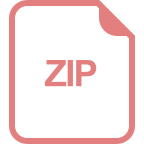
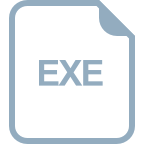
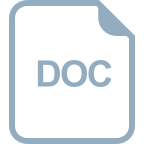
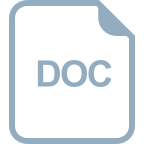
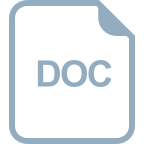
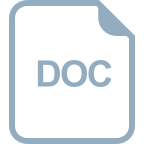
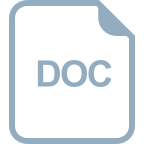