假设你精通C++ QT,帮我实现整张bmp格式图片显示在界面上不能有边框差距,像素要对应上
时间: 2024-03-13 20:43:02 浏览: 86
好的,下面是一个实现bmp图片显示的简单示例代码。该代码使用QT中的QImage类来加载bmp图片文件,并将其显示在一个QLabel控件中。请注意,bmp图片格式的处理需要考虑字节对齐等问题,因此下面的代码使用了一些特殊的处理方式来确保图片像素对应正确。
```c++
#include <QImage>
#include <QLabel>
#include <QVBoxLayout>
#include <QFile>
int main(int argc, char *argv[])
{
Q_UNUSED(argc);
Q_UNUSED(argv);
// 创建一个QWidget窗口
QWidget *widget = new QWidget();
widget->setWindowTitle("BMP Viewer");
// 创建一个QLabel控件,用于显示bmp图片
QLabel *label = new QLabel(widget);
label->setAlignment(Qt::AlignCenter);
// 创建一个QVBoxLayout布局管理器,并将QLabel控件添加到其中
QVBoxLayout *layout = new QVBoxLayout(widget);
layout->addWidget(label);
// 打开bmp图片文件
QFile file("test.bmp");
if (!file.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open file";
return -1;
}
// 读取bmp图片文件头
BITMAPFILEHEADER fileHeader;
if (file.read(reinterpret_cast<char*>(&fileHeader), sizeof(BITMAPFILEHEADER)) != sizeof(BITMAPFILEHEADER)) {
qDebug() << "Failed to read file header";
return -1;
}
// 读取bmp图片信息头
BITMAPINFOHEADER infoHeader;
if (file.read(reinterpret_cast<char*>(&infoHeader), sizeof(BITMAPINFOHEADER)) != sizeof(BITMAPINFOHEADER)) {
qDebug() << "Failed to read info header";
return -1;
}
// 计算bmp图片每行的字节数
int bytesPerLine = (infoHeader.biWidth * infoHeader.biBitCount + 31) / 32 * 4;
// 分配内存保存bmp图片像素数据
int imageSize = bytesPerLine * infoHeader.biHeight;
uchar* data = new uchar[imageSize];
// 读取bmp图片像素数据
if (file.read(reinterpret_cast<char*>(data), imageSize) != imageSize) {
qDebug() << "Failed to read image data";
delete[] data;
return -1;
}
// 将bmp图片像素数据转换为QImage格式
QImage image(data, infoHeader.biWidth, infoHeader.biHeight, QImage::Format_RGB888);
// 将QImage显示在QLabel控件中
label->setPixmap(QPixmap::fromImage(image));
// 调整QWidget窗口大小,以适应QLabel控件的尺寸
widget->resize(label->pixmap()->size());
// 显示QWidget窗口
widget->show();
// 释放内存
delete[] data;
return 0;
}
```
需要注意的是,bmp图片格式的处理可能比较麻烦,因为它的像素排列方式涉及到字节对齐等问题。因此,在实际应用中,需要根据具体的需求进行调整和完善。
阅读全文
相关推荐





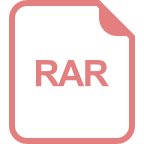



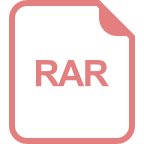






