编写一个函数,计算任意两个数字之间所能组成的奇数个数,数字必须是个位数
时间: 2023-04-27 07:05:39 浏览: 106
以下是Python代码实现:
def odd_numbers_between(num1, num2):
count = 0
for i in range(num1, num2+1):
for j in range(num1, num2+1):
if i != j and (i+j) % 2 != 0:
count += 1
return count
其中,num1和num2分别为两个数字,函数返回它们之间所能组成的奇数个数。函数的实现思路是,通过两个循环枚举所有可能的数字组合,判断它们的和是否为奇数,如果是,则计数器加1。最后返回计数器的值即可。
相关问题
编写一个函数来计算各个数字之和 C语言
在C语言中,编写一个函数来计算给定数组中所有数字的和是非常常见的练习。这里是一个简单的示例,展示如何创建一个名为`sum_array`的函数:
```c
#include <stdio.h>
// 定义函数,接收一个整数数组和其大小作为参数
int sum_array(int arr[], int n) {
int total = 0; // 初始化总和为0
// 遍历整个数组
for (int i = 0; i < n; i++) {
total += arr[i]; // 将当前元素添加到总和中
}
return total; // 返回数组的总和
}
int main() {
int numbers[] = {1, 2, 3, 4, 5}; // 示例数组
int size = sizeof(numbers) / sizeof(numbers[0]); // 计算数组长度
// 调用函数并打印结果
int sum = sum_array(numbers, size);
printf("The sum of the array elements is: %d\n", sum);
return 0;
}
```
在这个例子中,`sum_array`函数接受一个整数数组`arr`和它的长度`n`,然后通过循环逐个累加数组元素。在`main`函数中,我们声明了一个数组并调用了这个函数来计算其和。
编写一个函数型M文件计算整数的各位数字的乘积
在Matlab中,编写一个函数型M文件(.m文件)来计算整数的各位数字的乘积,可以创建一个名为`digit_product.m`的文件,内容如下:
```matlab
function result = digit_product(input_number)
% 检查输入是否为正整数
if ~isscalar(input_number) || ~isnumeric(input_number) || input_number < 0
error('Input must be a non-negative integer.');
end
% 将整数转换为字符串,以便按字符处理每个数字
str_num = num2str(input_number);
% 初始化乘积为1
product = 1;
% 遍历字符串中的每一位
for i = 1:length(str_num)
% 获取当前位的数字
current_digit = str_num(i);
% 确保只有数字参与乘积计算
if isnumeric(current_digit)
product *= current_digit;
end
end
% 返回结果
result = product;
end
```
你可以像这样调用这个函数:
```matlab
input = 12345; % 示例输入
result = digit_product(input);
disp(['The product of the digits is: ', num2str(result)]);
```
阅读全文
相关推荐
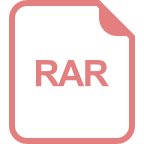
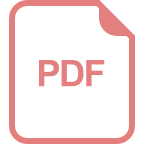
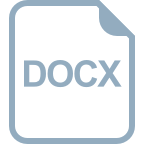













