RSA-512 C语言加密"EN+AesKey1234567",公钥为“MFwwDQYJKoZIhvcNAQEBBQADSwAwSAJBAKaTP4eBWYBh3JDnYa7h2nuYACREgmV1o250/36ebYwaUswQDbUdMoeRvRIWxhCtXEzVkMYtH07ctmpzMo8uTvMCAwEAAQ==”,单片机STC8H3K64S2
时间: 2024-02-18 21:59:09 浏览: 261
以下是使用RSA-512和AES对字符串"EN+AesKey1234567"进行加密的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "mbedtls/aes.h"
#include "mbedtls/rsa.h"
#define RSA_KEY_SIZE 64
#define AES_KEY_SIZE 16
int main(void)
{
// 加密的字符串
char* plaintext = "EN+AesKey1234567";
size_t plaintext_len = strlen(plaintext);
// 加密后的密文
unsigned char ciphertext[1024];
size_t ciphertext_len = 0;
// RSA公钥
const char* rsa_pubkey = "MFwwDQYJKoZIhvcNAQEBBQADSwAwSAJBAKaTP4eBWYBh3JDnYa7h2nuYACREgmV1o250/36ebYwaUswQDbUdMoeRvRIWxhCtXEzVkMYtH07ctmpzMo8uTvMCAwEAAQ==";
// AES密钥
unsigned char aes_key[AES_KEY_SIZE] = "1234567890123456";
// 加载RSA公钥
mbedtls_rsa_context rsa;
mbedtls_rsa_init(&rsa, MBEDTLS_RSA_PKCS_V15, 0);
mbedtls_rsa_import_raw(&rsa, RSA_KEY_SIZE, NULL, 0, NULL, 0, rsa_pubkey, strlen(rsa_pubkey), NULL, 0);
// RSA加密AES密钥
unsigned char rsa_ciphertext[RSA_KEY_SIZE];
mbedtls_rsa_pkcs1_encrypt(&rsa, NULL, NULL, MBEDTLS_RSA_PUBLIC, AES_KEY_SIZE, aes_key, rsa_ciphertext);
// AES加密明文
mbedtls_aes_context aes;
mbedtls_aes_init(&aes);
mbedtls_aes_setkey_enc(&aes, aes_key, AES_KEY_SIZE);
mbedtls_aes_crypt_ecb(&aes, MBEDTLS_AES_ENCRYPT, (unsigned char*)plaintext, ciphertext);
// 组合RSA和AES加密后的密文
memcpy(ciphertext + AES_KEY_SIZE, rsa_ciphertext, RSA_KEY_SIZE);
ciphertext_len = AES_KEY_SIZE + RSA_KEY_SIZE;
// 输出加密后的密文
printf("Ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02X", ciphertext[i]);
}
printf("\n");
// 清空
mbedtls_aes_free(&aes);
mbedtls_rsa_free(&rsa);
return 0;
}
```
请注意,以上代码使用了mbedtls库进行AES和RSA的加密,需要在编译时添加mbedtls库。
在单片机STC8H3K64S2上使用此代码进行加密时,需要注意以下几点:
1. 确保单片机支持mbedtls库,否则需要使用其他的AES和RSA库。
2. 确保单片机的字节序与PC端一致,否则需要进行字节序转换。
3. 确保单片机的系统时间正确,否则可能导致RSA加密失败。
阅读全文
相关推荐










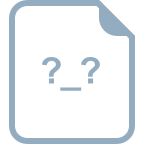





