#include <iostream> using namespace std; // 集合类的操作接口 class Collection { public: // 向集合中添加一个元素 virtual void add(int e) = 0; // 获取指定位置的元素 virtual int get(unsigned int i) const = 0; }; // 实现了集合接口 class Array : public Collection { public: Array(unsigned int s) { //**********found********** a = new ________________; size = s; num = 0; } ~Array() { //**********found********** _______________; } virtual void add(int e) { if (num < size) { //**********found********** _______________ = e; num++; } } virtual int get(unsigned int i) const { if (i < size) { //**********found********** _______________; } return 0; } private: int *a; unsigned int size; unsigned int num; }; void fun(Collection& col) { int i; for (i = 0; i < 10; i++) { col.add(i+1); } for (i = 0; i < 10; i++) { cout << col.get(i) << ", "; } cout << endl; } int main() { Array a(0xff); fun(a); return 0; }
时间: 2023-06-29 15:16:24 浏览: 107
答案如下:
```c++
a = new int[size];
```
```c++
delete[] a;
```
```c++
a[num] = e;
```
```c++
return a[i];
```
这段代码定义了一个集合类的操作接口 `Collection`,其中包含了向集合中添加一个元素和获取指定位置的元素两个纯虚函数。然后又定义了一个实现了集合接口的类 `Array`,它使用了动态数组来存储元素,并实现了接口中的两个函数。在 `main` 函数中,我们创建了一个 `Array` 对象,并通过一个引用调用 `fun` 函数。`fun` 函数调用了 `Collection` 接口中的函数,而实际上使用的是 `Array` 类中的函数。最终程序输出了 `1, 2, 3, 4, 5, 6, 7, 8, 9, 10,`。
相关问题
设计支持集合操作的类模板,适合数值类型,设计适当的成员函数,实现支持添加,删除元素,求和,求平均值,查找,排序。在主函数创建对象,调用已完成的函数。
好的,我了解您的需求。下面是一个支持集合操作的类模板的示例代码:
```c++
#include <iostream>
#include <vector>
<algorithm>
using namespace std;
template <typename Tclass Collection {
public:
Collection() {}
void add(T value) {
elements.push_back(value);
}
void remove(T value) {
elements.erase(remove(elements.begin(), elements.end(), value), elements.end());
}
T sum() {
T sum = 0;
for (auto it = elements.begin(); it != elements.end(); it++) {
sum += *it;
}
return sum;
}
double average() {
if (elements.empty()) {
return 0;
}
return static_cast<double>(sum()) / elements.size();
}
bool contains(T value) {
return find(elements.begin(), elements.end(), value) != elements.end();
}
void sort() {
std::sort(elements.begin(), elements.end());
}
private:
vector<T> elements;
};
int main() {
Collection<int> c;
c.add(1);
c.add(2);
c.add(3);
cout << "Sum: " << c.sum() << endl;
cout << "Average: " << c.average() << endl;
cout << "Contains 2: " << c.contains(2) << endl;
c.sort();
cout << "Sorted: ";
for (auto it = c.begin(); it != c.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
在上面的示例代码中,我们使用了一个 vector 来存储元素。add() 函数用于添加元素,remove() 函数用于删除元素。sum() 函数用于求和,average() 函数用于求平均值。contains() 函数用于查找元素是否存在,sort() 函数用于排序。
在主函数中,我们创建了一个 Collection<int> 对象,并向其中添加了一些元素。然后,我们调用了一些成员函数,并输出了结果。最后,我们对集合进行了排序,并输出了排序后的结果。
希望这个示例代码能够帮到您!
1.设计支持集合操作的类模板,适合数值类型,设计适当的成员函数,实现支持添加,删除元素,求和,求平均值,查找,排序。在主函数创建对象,调用已完成的函数。
好的,我明白了。下面是一个支持集合操作的类模板的示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
template <typename T>
class Collection {
public:
Collection() {}
void add(T value) {
elements.push_back(value);
}
void remove(T value) {
elements.erase(remove(elements.begin(), elements.end(), value), elements.end());
}
T sum() {
T sum = 0;
for (auto it = elements.begin(); it != elements.end(); it++) {
sum += *it;
}
return sum;
}
double average() {
if (elements.empty()) {
return 0;
}
return static_cast<double>(sum()) / elements.size();
}
bool contains(T value) {
return find(elements.begin(), elements.end(), value) != elements.end();
}
void sort() {
std::sort(elements.begin(), elements.end());
}
private:
vector<T> elements;
};
int main() {
Collection<int> c;
c.add(1);
c.add(2);
c.add(3);
cout << "Sum: " << c.sum() << endl;
cout << "Average: " << c.average() << endl;
cout << "Contains 2: " << c.contains(2) << endl;
c.sort();
cout << "Sorted: ";
for (auto it = c.begin(); it != c.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
上面的示例代码中,我们使用了一个 vector 来存储元素。add() 函数用于添加元素,remove() 函数用于删除元素。sum() 函数用于求和,average() 函数用于求平均值。contains() 函数用于查找元素是否存在,sort() 函数用于排序。
在主函数中,我们创建了一个 Collection<int> 对象,并向其中添加了一些元素。然后,我们调用了一些成员函数,并输出了结果。最后,我们对集合进行了排序,并输出了排序后的结果。
希望这个示例代码能够帮到您!
阅读全文
相关推荐
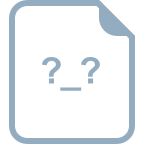
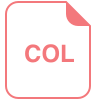
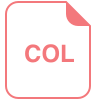
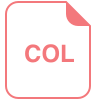
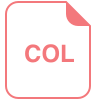
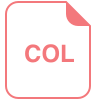
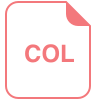
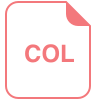


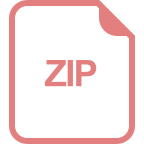
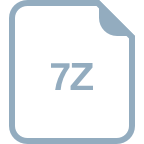