springboot中使用aop监听注解
时间: 2024-02-09 12:09:49 浏览: 98
在Spring Boot中使用AOP监听注解可以通过以下步骤实现:
1. 在`pom.xml`文件中添加`spring-boot-starter-aop`依赖,以使用Spring AOP。
2. 创建一个注解类,例如`@MyAnnotation`,用于标记需要被监听的方法。
3. 创建一个切面类,用于监听被`@MyAnnotation`标记的方法。可以使用`@Aspect`注解来标记这个类。
4. 在切面类中定义一个切点,用于匹配被`@MyAnnotation`标记的方法。可以使用`@Pointcut`注解来定义切点。
5. 在切面类中定义一个通知,用于在匹配到切点时执行某些操作。可以使用`@Before`、`@After`、`@Around`等注解来定义通知。
6. 在Spring Boot应用程序的主类中添加`@EnableAspectJAutoProxy`注解,以启用AOP功能。
以下是一个示例代码:
```java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyAnnotation {
}
@Aspect
@Component
public class MyAspect {
@Pointcut("@annotation(com.example.demo.MyAnnotation)")
public void myAnnotationPointcut() {}
@Before("myAnnotationPointcut()")
public void beforeMyAnnotation() {
System.out.println("Before My Annotation");
}
}
@EnableAspectJAutoProxy
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
在上面的示例中,`@MyAnnotation`注解用于标记需要被监听的方法,在`MyAspect`切面类中定义了一个切点`myAnnotationPointcut`,用于匹配被`@MyAnnotation`标记的方法,在`beforeMyAnnotation`方法中定义了一个前置通知,在匹配到切点时执行。在`DemoApplication`主类中添加了`@EnableAspectJAutoProxy`注解,以启用AOP功能。
阅读全文
相关推荐

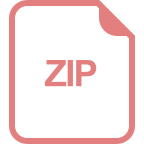
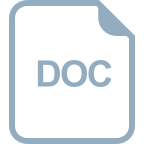
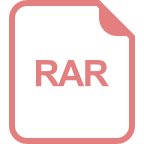
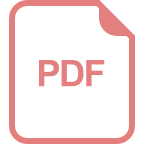
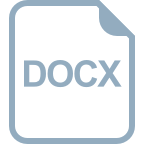
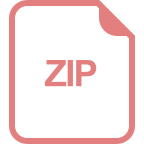
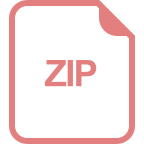
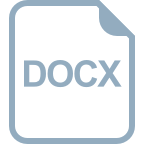
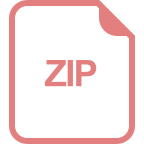
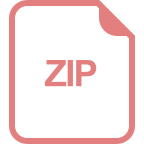
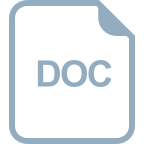
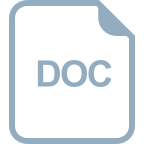
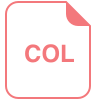

