网络服务能力测试工具的详细设计与实现---网络包的获取 C/C++
时间: 2023-12-03 08:46:42 浏览: 98
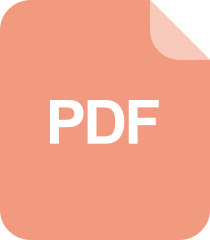
用C实现网络编程---抓取网络数据包的实现方法.pdf
获取网络包的过程需要使用到C/C++中的socket编程,主要包括以下步骤:
1. 创建socket套接字
```c++
int sockfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sockfd < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
```
2. 绑定网卡接口
```c++
struct ifreq ifr;
memset(&ifr, 0, sizeof(ifr));
strncpy(ifr.ifr_name, "eth0", IFNAMSIZ - 1);
if (setsockopt(sockfd, SOL_SOCKET, SO_BINDTODEVICE, (void*)&ifr, sizeof(ifr)) < 0) {
perror("setsockopt");
close(sockfd);
exit(EXIT_FAILURE);
}
```
3. 接收网络包
```c++
ssize_t n;
unsigned char buf[2048];
n = recvfrom(sockfd, buf, sizeof(buf), 0, NULL, NULL);
if (n < 0) {
perror("recvfrom");
close(sockfd);
exit(EXIT_FAILURE);
}
```
4. 解析网络包
网络包的解析需要根据具体协议进行不同的处理,例如以太网帧、IP数据报、TCP/UDP数据报等。以太网帧的解析可以使用以下代码:
```c++
struct ethhdr *eth = (struct ethhdr*)buf;
printf("Ethernet header:\n");
printf(" Destination MAC address: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth->h_dest[0], eth->h_dest[1], eth->h_dest[2], eth->h_dest[3], eth->h_dest[4], eth->h_dest[5]);
printf(" Source MAC address: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth->h_source[0], eth->h_source[1], eth->h_source[2], eth->h_source[3], eth->h_source[4], eth->h_source[5]);
printf(" Protocol type: %04x\n", ntohs(eth->h_proto));
```
以上代码可以得到以太网帧的目的MAC地址、源MAC地址和协议类型。
综上所述,获取网络包的过程主要包括创建socket套接字、绑定网卡接口、接收网络包和解析网络包。根据具体的需求可以进行相应的修改和扩展。
阅读全文
相关推荐

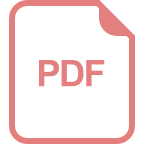
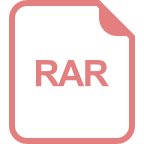
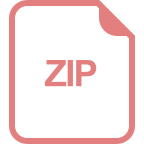
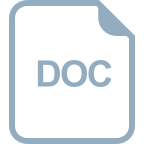
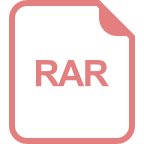
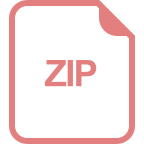
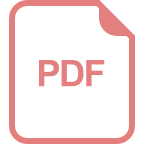
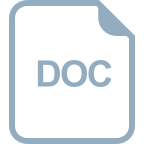
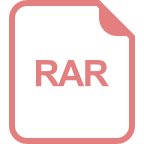
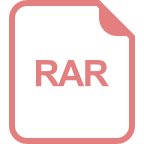
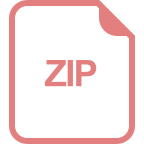
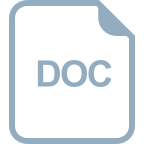
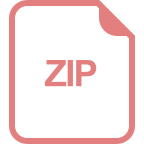
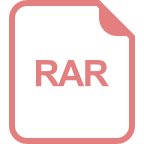
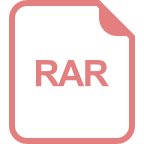