1. 生成两组线性均匀分布的数据(完全线性可分)。 生成两组线性均匀分布的数据(线性不可分)。 生成两组高斯分布的数据(完全线性可分)。 生成两组高斯分布的数据(线性不可分)。 2. 使用 神经网络-感知器进行二分类,并画出分类界面。python
时间: 2024-02-09 13:12:52 浏览: 65
好的,以下是 Python 代码实现:
1. 生成数据:
```python
import numpy as np
# 生成两组线性均匀分布的数据(完全线性可分)
# 第一组数据
np.random.seed(1)
X1 = np.random.uniform(-1, 1, (100, 2))
y1 = np.ones(100)
# 第二组数据
X2 = np.random.uniform(-1, 1, (100, 2)) + np.array([2, 2])
y2 = np.zeros(100)
# 生成两组线性均匀分布的数据(线性不可分)
# 第一组数据
np.random.seed(1)
X1 = np.random.uniform(-1, 1, (100, 2))
y1 = np.ones(100)
# 第二组数据
X2 = np.random.uniform(-1, 1, (100, 2))
y2 = np.zeros(100)
# 将第二组数据分布到一个圆形区域内
X2_norm = np.sqrt(np.sum(X2**2, axis=1))
X2 = X2[X2_norm <= 0.8, :]
y2 = y2[X2_norm <= 0.8]
# 生成两组高斯分布的数据(完全线性可分)
# 第一组数据
np.random.seed(1)
X1 = np.random.normal(0, 1, (100, 2))
y1 = np.ones(100)
# 第二组数据
X2 = np.random.normal(3, 1, (100, 2))
y2 = np.zeros(100)
# 生成两组高斯分布的数据(线性不可分)
# 第一组数据
np.random.seed(1)
X1 = np.random.normal(0, 1, (100, 2))
y1 = np.ones(100)
# 第二组数据
X2 = np.random.normal(0, 1, (100, 2))
y2 = np.zeros(100)
# 将第二组数据分布到一个圆形区域内
X2_norm = np.sqrt(np.sum(X2**2, axis=1))
X2 = X2[X2_norm <= 0.8, :]
y2 = y2[X2_norm <= 0.8]
```
2. 使用神经网络-感知器进行二分类,并画出分类界面:
```python
from sklearn.linear_model import Perceptron
from sklearn.svm import SVC
import matplotlib.pyplot as plt
# 完全线性可分的数据集
# 使用 Perceptron 模型进行分类,并画出分类界面
perceptron = Perceptron()
perceptron.fit(np.vstack((X1, X2)), np.hstack((y1, y2)))
w = perceptron.coef_[0]
b = perceptron.intercept_[0]
x_min, x_max = np.vstack((X1, X2))[:, 0].min() - 1, np.vstack((X1, X2))[:, 0].max() + 1
y_min, y_max = np.vstack((X1, X2))[:, 1].min() - 1, np.vstack((X1, X2))[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02), np.arange(y_min, y_max, 0.02))
Z = perceptron.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=plt.cm.Paired)
plt.scatter(X1[:, 0], X1[:, 1], c='r')
plt.scatter(X2[:, 0], X2[:, 1], c='b')
plt.show()
# 线性不可分的数据集
# 使用 SVC 模型进行非线性变换,并使用 Perceptron 模型进行分类,画出分类界面
svc = SVC(kernel='rbf', gamma=0.7, C=1)
svc.fit(np.vstack((X1, X2)), np.hstack((y1, y2)))
X_train = svc.transform(np.vstack((X1, X2)))
y_train = np.hstack((y1, y2))
perceptron = Perceptron()
perceptron.fit(X_train, y_train)
x_min, x_max = X_train[:, 0].min() - 1, X_train[:, 0].max() + 1
y_min, y_max = X_train[:, 1].min() - 1, X_train[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02), np.arange(y_min, y_max, 0.02))
Z = perceptron.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=plt.cm.Paired)
plt.scatter(X_train[:, 0], X_train[:, 1], c=y_train)
plt.show()
```
阅读全文
相关推荐
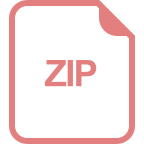
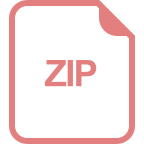





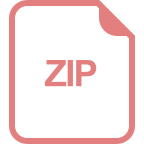
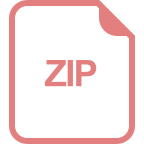
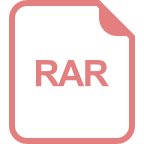
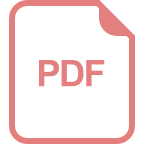
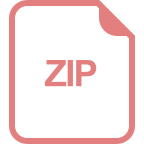
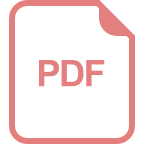
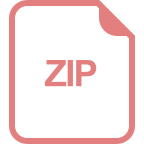