如何用java写一个随机生成迷宫的难度可调的有动画的迷宫鼠程序
时间: 2023-12-03 10:44:13 浏览: 116
以下是一个使用Java编写的随机生成迷宫的程序,其中包含可调整难度的选项和动画效果:
```java
import java.awt.*;
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
public class MazeGenerator extends JPanel implements ActionListener {
private static final int ROWS = 25;
private static final int COLUMNS = 25;
private static final int CELL_SIZE = 20;
private static final int DELAY = 50;
private boolean[][] cellsVisited = new boolean[ROWS][COLUMNS];
private boolean[][] wallsHorizontal = new boolean[ROWS + 1][COLUMNS];
private boolean[][] wallsVertical = new boolean[ROWS][COLUMNS + 1];
private Random random = new Random();
private Timer timer;
private int delay;
public MazeGenerator(int delay) {
this.delay = delay;
setBackground(Color.BLACK);
setPreferredSize(new Dimension(COLUMNS * CELL_SIZE, ROWS * CELL_SIZE));
setDoubleBuffered(true);
timer = new Timer(delay, this);
timer.start();
}
public void actionPerformed(ActionEvent e) {
generateMaze();
repaint();
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
drawMaze(g);
}
private void generateMaze() {
int currentRow = random.nextInt(ROWS);
int currentColumn = random.nextInt(COLUMNS);
cellsVisited[currentRow][currentColumn] = true;
while (true) {
ArrayList<Point> neighbors = new ArrayList<Point>();
if (currentRow > 0 && !cellsVisited[currentRow - 1][currentColumn]) {
neighbors.add(new Point(currentRow - 1, currentColumn));
}
if (currentRow < ROWS - 1 && !cellsVisited[currentRow + 1][currentColumn]) {
neighbors.add(new Point(currentRow + 1, currentColumn));
}
if (currentColumn > 0 && !cellsVisited[currentRow][currentColumn - 1]) {
neighbors.add(new Point(currentRow, currentColumn - 1));
}
if (currentColumn < COLUMNS - 1 && !cellsVisited[currentRow][currentColumn + 1]) {
neighbors.add(new Point(currentRow, currentColumn + 1));
}
if (neighbors.size() == 0) {
break;
}
Point chosenNeighbor = neighbors.get(random.nextInt(neighbors.size()));
int chosenRow = chosenNeighbor.x;
int chosenColumn = chosenNeighbor.y;
if (chosenRow == currentRow - 1) {
wallsHorizontal[currentRow][currentColumn] = true;
}
else if (chosenRow == currentRow + 1) {
wallsHorizontal[currentRow + 1][currentColumn] = true;
}
else if (chosenColumn == currentColumn - 1) {
wallsVertical[currentRow][currentColumn] = true;
}
else if (chosenColumn == currentColumn + 1) {
wallsVertical[currentRow][currentColumn + 1] = true;
}
currentRow = chosenRow;
currentColumn = chosenColumn;
cellsVisited[currentRow][currentColumn] = true;
try {
Thread.sleep(DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void drawMaze(Graphics g) {
g.setColor(Color.WHITE);
for (int i = 0; i <= ROWS; i++) {
for (int j = 0; j <= COLUMNS; j++) {
if (wallsHorizontal[i][j]) {
g.drawLine(j * CELL_SIZE, i * CELL_SIZE, (j + 1) * CELL_SIZE, i * CELL_SIZE);
}
if (wallsVertical[i][j]) {
g.drawLine(j * CELL_SIZE, i * CELL_SIZE, j * CELL_SIZE, (i + 1) * CELL_SIZE);
}
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Maze Generator");
MazeGenerator maze = new MazeGenerator(DELAY);
frame.add(maze);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
这个程序使用Java Swing库来绘制迷宫,其中包含一个可调整的延迟参数,用于控制动画速度和难度。程序使用深度优先搜索算法来生成迷宫,每次随机选择一个未访问的邻居作为下一个位置,并在生成墙壁时使用延迟来创建动画效果。最终的迷宫是由水平和垂直墙壁组成的网格,可以通过绘制线条来实现。
阅读全文
相关推荐
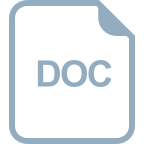
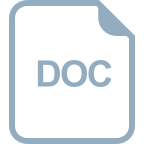
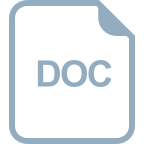
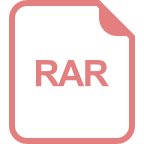
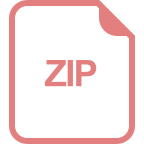
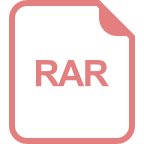
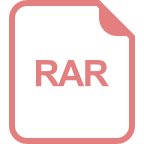
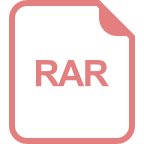
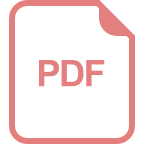
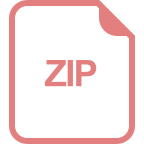
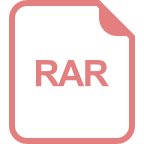
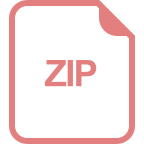
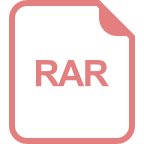
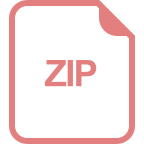
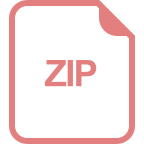
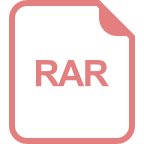
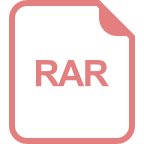