stream().sorted的使用案列
时间: 2023-10-13 14:20:36 浏览: 69
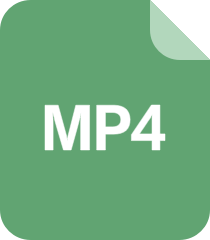
10.第十节-使用stream流sorted实现排序.mp4
stream().sorted是Java 8中Stream API的一个方法,它可以对流中的元素进行排序。它返回一个新的流,其中元素按自然顺序排序,或者按指定的Comparator排序。
下面是一个使用案例:
假设有一个学生类Student,包含学生的姓名和年龄信息,我们需要按年龄对学生进行排序。
```java
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Tom", 20));
students.add(new Student("Jerry", 18));
students.add(new Student("Lucy", 22));
// 按年龄升序排序
List<Student> sortedStudents = students.stream().sorted(Comparator.comparing(Student::getAge)).toList();
System.out.println(sortedStudents);
// 按年龄降序排序
sortedStudents = students.stream().sorted(Comparator.comparing(Student::getAge).reversed()).toList();
System.out.println(sortedStudents);
}
}
```
输出结果如下:
```
[Student{name='Jerry', age=18}, Student{name='Tom', age=20}, Student{name='Lucy', age=22}]
[Student{name='Lucy', age=22}, Student{name='Tom', age=20}, Student{name='Jerry', age=18}]
```
可以看到,我们使用了sorted方法对学生列表进行了排序,并且可以通过Comparator来指定排序规则。
阅读全文
相关推荐
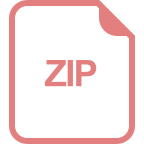
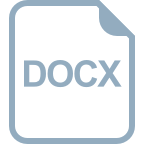















