利用python TensorFlow训练语言模型代码
时间: 2023-11-22 18:54:52 浏览: 34
以下是使用TensorFlow训练语言模型的示例代码:
首先,需要导入必要的库:
```python
import tensorflow as tf
import numpy as np
```
然后,我们定义一些训练数据和超参数:
```python
# 定义训练数据
corpus = "This is a sample text. You can replace it with your own data."
# 将文本转换成小写并分割成单词列表
words = corpus.lower().split()
# 定义超参数
vocab_size = len(set(words))
embedding_dim = 64
hidden_dim = 128
num_epochs = 100
batch_size = 32
learning_rate = 0.001
```
接下来,我们需要将文本数据转换成可供模型训练的形式。我们首先创建一个单词到索引的映射字典,并将所有单词转换成对应的索引:
```python
# 创建单词到索引的映射字典
word2idx = {word: i for i, word in enumerate(set(words))}
# 将所有单词转换成对应的索引
data = [word2idx[word] for word in words]
```
然后,我们定义模型的输入和输出,并创建模型:
```python
# 定义模型的输入和输出
inputs = tf.keras.layers.Input(shape=(None,))
embed = tf.keras.layers.Embedding(vocab_size, embedding_dim)(inputs)
lstm = tf.keras.layers.LSTM(hidden_dim)(embed)
outputs = tf.keras.layers.Dense(vocab_size, activation='softmax')(lstm)
# 创建模型
model = tf.keras.Model(inputs, outputs)
```
现在,我们可以定义损失函数和优化器,并编译模型:
```python
# 定义损失函数和优化器
loss_fn = tf.keras.losses.SparseCategoricalCrossentropy()
optimizer = tf.keras.optimizers.Adam(learning_rate)
# 编译模型
model.compile(loss=loss_fn, optimizer=optimizer)
```
接下来,我们可以定义一个生成批次数据的函数:
```python
def generate_batches(data, batch_size):
num_batches = len(data) // batch_size
for i in range(num_batches):
batch = data[i * batch_size:(i + 1) * batch_size]
x = batch[:-1]
y = batch[1:]
yield (np.array(x), np.array(y))
```
现在,我们可以使用生成器训练模型:
```python
# 训练模型
for epoch in range(num_epochs):
print("Epoch:", epoch + 1)
for x, y in generate_batches(data, batch_size):
loss = model.train_on_batch(x, y)
print("Batch loss:", loss)
```
最后,我们可以使用训练好的模型生成新文本:
```python
# 使用训练好的模型生成新文本
start_word = "this"
num_words = 10
for _ in range(num_words):
x = np.array([[word2idx[start_word]]])
pred = model.predict(x)[0]
idx = np.argmax(pred)
next_word = list(word2idx.keys())[list(word2idx.values()).index(idx)]
start_word += " " + next_word
print(start_word)
```
这就是使用TensorFlow训练语言模型的基本流程。当然,具体的实现方式会因为不同的需求而有所不同。
相关推荐
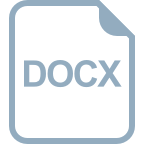
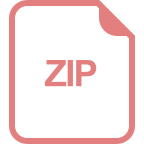
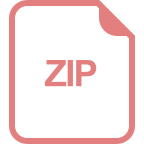
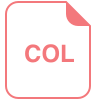
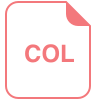
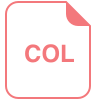
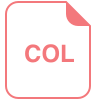
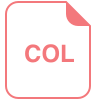









