c语言编写程序将两个字符串连接,不要用stract函数。
时间: 2024-05-09 15:18:34 浏览: 114
可以使用循环遍历字符串,将第一个字符串的每个字符依次复制到新字符串中,再将第二个字符串的每个字符也依次复制到新字符串末尾即可实现字符串连接。具体的实现可以参考以下代码:
```
#include <stdio.h>
#include <stdlib.h>
int main()
{
char str1[100], str2[100], new_str[200];
int i, j;
printf("请输入第一个字符串:");
scanf("%s", str1);
printf("请输入第二个字符串:");
scanf("%s", str2);
// 将第一个字符串的字符复制到新字符串中
for (i = 0; str1[i] != '\0'; i++) {
new_str[i] = str1[i];
}
// 将第二个字符串的字符复制到新字符串末尾
for (j = 0; str2[j] != '\0'; j++) {
new_str[i + j] = str2[j];
}
// 添加字符串结束标志
new_str[i + j] = '\0';
printf("连接后的字符串为:%s\n", new_str);
return 0;
}
```
相关问题
将两个字符串连接,不用stract函数 C语言
可以使用字符数组和指针来实现字符串的连接,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
char str1[20] = "hello";
char str2[20] = "world";
char *result;
result = (char *)malloc(strlen(str1) + strlen(str2) + 1); // 申请空间
if (result == NULL) // 判断是否申请成功
{
printf("Memory allocation failed.\n");
exit(1);
}
strcpy(result, str1); // 拷贝第一个字符串
strcat(result, str2); // 连接第二个字符串
printf("The result of string concatenation is: %s\n", result);
free(result); // 释放内存
return 0;
}
```
输出结果为:
```
The result of string concatenation is: helloworld
```
用c语言编写:将两个字符串连接起来,不使用stract()函数
可以使用指针操作和循环来将两个字符串连接起来。以下是一个简单的示例程序,将字符串s1和s2连接起来:
```c
#include <stdio.h>
int main()
{
char s1[100], s2[100];
int i, j;
printf("Enter the first string: ");
scanf("%s", s1);
printf("Enter the second string: ");
scanf("%s", s2);
// Find the end of the first string
i = 0;
while (s1[i] != '\0') {
i++;
}
// Append the second string to the first string
j = 0;
while (s2[j] != '\0') {
s1[i] = s2[j];
i++;
j++;
}
// Terminate the string with a null character
s1[i] = '\0';
printf("The concatenated string is: %s\n", s1);
return 0;
}
```
在此示例程序中,我们首先使用scanf()函数从用户输入中读取两个字符串s1和s2。然后,我们使用一个while循环找到字符串s1的末尾(即null字符'\0'之前的最后一个字符的索引)。然后,我们使用另一个while循环将字符串s2附加到字符串s1的末尾。最后,我们在新字符串的末尾添加了一个null字符,以将其作为C字符串的终止符。
请注意,这只是一个简单的示例,可能会有一些边缘情况需要处理(例如,如果输入的字符串长度超过了s1和s2数组的大小)。
阅读全文
相关推荐
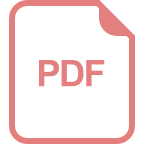





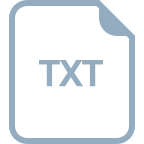
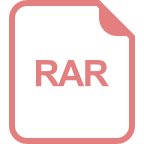
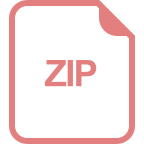