restTemplate 发送http post请求带有文件流、参数
时间: 2024-02-01 14:04:21 浏览: 37
可以使用`RestTemplate`的`postForEntity`方法来发送HTTP POST请求,同时携带文件流和其他参数。具体可以参考下面的示例代码:
```java
import org.springframework.core.io.ByteArrayResource;
import org.springframework.core.io.Resource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class RestClient {
public static void main(String[] args) throws IOException {
String url = "http://example.com/upload";
// 设置请求头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
// 设置请求体
MultiValueMap<String, Object> body = new LinkedMultiValueMap<>();
// 添加文件流
Path filePath = Paths.get("path/to/file.txt");
byte[] fileBytes = Files.readAllBytes(filePath);
Resource fileResource = new ByteArrayResource(fileBytes) {
@Override
public String getFilename() {
return filePath.getFileName().toString();
}
};
body.add("file", fileResource);
// 添加其他参数
body.add("param1", "value1");
body.add("param2", "value2");
HttpEntity<MultiValueMap<String, Object>> requestEntity = new HttpEntity<>(body, headers);
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.postForEntity(url, requestEntity, String.class);
System.out.println(response.getBody());
}
}
```
在上面的示例代码中,我们首先设置了请求头,指定了请求体的类型为`multipart/form-data`。然后构造了一个`MultiValueMap`对象,用于存储请求体的键值对。通过`ByteArrayResource`类可以将文件流转化为Spring的`Resource`对象,并将其添加到请求体中。最后,我们使用`postForEntity`方法发送HTTP POST请求,并将响应的内容解析为字符串。
相关推荐
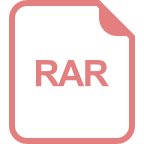














