请用Java语言写一个快速排序
时间: 2023-08-25 18:09:27 浏览: 101
### 回答1:
我可以为您提供一个简单的快速排序示例:
public class QuickSort {
public static void quickSort(int[] arr, int start, int end) {
int partition = partition(arr, start, end);
if(partition-1>start) {
quickSort(arr, start, partition - 1);
}
if(partition+1<end) {
quickSort(arr, partition + 1, end);
}
}
public static int partition(int[] arr, int start, int end) {
int pivot = arr[end];
for(int i=start; i<end; i++) {
if(arr[i]<pivot) {
int temp= arr[start];
arr[start]=arr[i];
arr[i]=temp;
start++;
}
}
int temp = arr[start];
arr[start] = pivot;
arr[end] = temp;
return start;
}
}
### 回答2:
快速排序是一种常用的排序算法,它通过重新排列要排序的元素,将待排序的序列分割成独立的两部分,其中一部分的所有元素都小于另一部分。接下来我将使用Java语言编写一个快速排序算法示例。
```java
public class QuickSort {
public static void main(String[] args) {
int[] arr = {5, 9, 3, 1, 8, 6, 2, 7, 4};
quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
}
```
以上示例代码中,我们首先定义了一个`QuickSort`类,并在`main`方法中初始化一个待排序的数组`arr`。然后我们调用`quickSort`方法对数组进行快速排序,并最后在控制台打印排序后的结果。
`quickSort`方法是快速排序的主要逻辑实现,它接受三个参数:待排序数组`arr`、开始位置`low`和结束位置`high`。在方法内部,我们首先判断`low`是否小于`high`,如果是,则调用`partition`方法对数组进行分区,并递归调用`quickSort`方法对左右两个子数组进行排序。
`partition`方法用于进行分区操作,它接受三个参数:待排序数组`arr`、开始位置`low`和结束位置`high`。在方法内部,我们首先将开始位置的元素作为基准值`pivot`,然后使用两个指针`low`和`high`分别指向开始位置和结束位置。通过一系列的交换操作,将小于基准值的元素放在其左边,大于基准值的元素放在右边,最后返回基准值的位置。
以上即为使用Java语言编写的快速排序算法示例。要使用该算法,只需将待排序的数组传入`quickSort`方法即可。
### 回答3:
快速排序是一种常用的排序算法,它的基本思想是通过递归将待排序的元素分为左右两部分,其中左半部分的元素都小于等于基准值,右半部分的元素都大于基准值,然后再对左右两部分进行递归排序。
下面是使用Java语言实现的快速排序的代码:
```java
public class QuickSort {
public static void quickSort(int[] arr, int left, int right) {
if (left < right) {
int pivotIndex = partition(arr, left, right); // 获取基准值的位置
quickSort(arr, left, pivotIndex - 1); // 对基准值左边的元素进行递归排序
quickSort(arr, pivotIndex + 1, right); // 对基准值右边的元素进行递归排序
}
}
public static int partition(int[] arr, int left, int right) {
int pivot = arr[left]; // 选择第一个元素作为基准值
int i = left, j = right;
while (i < j) {
// 从右往左找第一个小于基准值的元素
while (i < j && arr[j] >= pivot) {
j--;
}
if (i < j) {
arr[i++] = arr[j];
}
// 从左往右找第一个大于等于基准值的元素
while (i < j && arr[i] < pivot) {
i++;
}
if (i < j) {
arr[j--] = arr[i];
}
}
arr[i] = pivot; // 将基准值放到正确的位置
return i;
}
public static void main(String[] args) {
int[] arr = {9, 5, 2, 7, 1, 6, 3, 8, 4};
quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
以上代码实现了快速排序算法,并通过一个简单的示例对数组 `{9, 5, 2, 7, 1, 6, 3, 8, 4}` 进行排序。输出结果为 `1 2 3 4 5 6 7 8 9`。
阅读全文
相关推荐
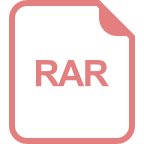
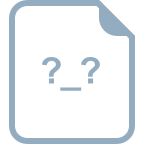
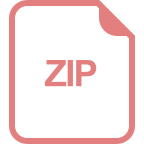
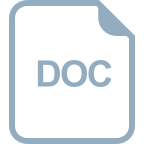
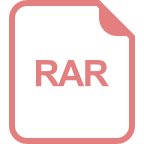
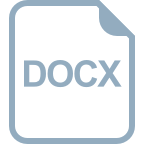
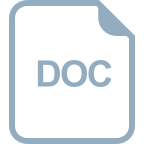
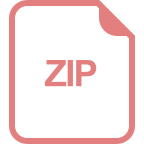
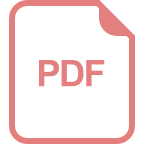
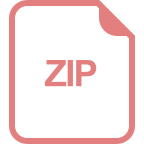
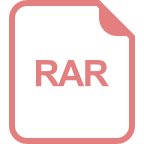