在arduino环境下使用ESP32C3芯片采用一个按钮控制电机正转反转与停止
时间: 2024-02-10 19:10:28 浏览: 64
好的,我可以为您解答这个问题。在上一个问题的基础上,我们可以使用一个变量来记录电机的状态,以便可以控制电机的停止。以下是具体步骤:
1. 定义一个变量来记录电机状态,例如:
```
int motorState = 0; // 0 表示电机停止,1 表示电机正转,-1 表示电机反转
```
2. 在按钮被按下时,根据电机的状态来执行相应的操作,例如:
```
if (motorState == 0) {
digitalWrite(motorPin, HIGH); // 电机正转
motorState = 1;
} else if (motorState == 1) {
digitalWrite(motorPin, LOW); // 电机停止
motorState = 0;
} else if (motorState == -1) {
digitalWrite(motorPin, HIGH); // 电机反转
motorState = -1;
}
```
3. 在按钮被释放时,停止电机:
```
if (buttonState == LOW) {
digitalWrite(motorPin, LOW); // 电机停止
motorState = 0;
}
```
以下是完整的示例代码:
```
// 定义按钮和电机引脚
const int buttonPin = 2;
const int motorPin = 3;
// 定义电机状态变量
int motorState = 0; // 0 表示电机停止,1 表示电机正转,-1 表示电机反转
void setup() {
// 初始化引脚
pinMode(buttonPin, INPUT);
pinMode(motorPin, OUTPUT);
}
void loop() {
// 读取按钮状态
int buttonState = digitalRead(buttonPin);
// 如果按钮被按下,则根据电机状态执行相应的操作
if (buttonState == HIGH) {
if (motorState == 0) {
digitalWrite(motorPin, HIGH); // 电机正转
motorState = 1;
} else if (motorState == 1) {
digitalWrite(motorPin, LOW); // 电机停止
motorState = 0;
} else if (motorState == -1) {
digitalWrite(motorPin, HIGH); // 电机反转
motorState = -1;
}
} else { // 如果按钮被释放,则停止电机
digitalWrite(motorPin, LOW); // 电机停止
motorState = 0;
}
}
```
同样需要注意,此代码仅为示例,并未经过实际测试。您需要根据自己的硬件配置和需求进行修改和调整。
阅读全文
相关推荐






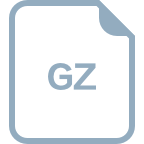








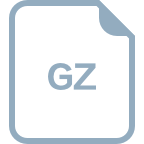