使用python调用C#生成的dll文件,调用dll的函数,将其封装为python函数,具体该如何实现
时间: 2024-03-15 09:45:36 浏览: 141
要使用Python调用C#生成的dll文件,可以使用Python的ctypes库。以下是具体实现步骤:
1. 首先需要在C#代码中将要调用的函数标记为“extern”,并使用DllImport属性导出该函数,如下所示:
```csharp
using System;
using System.Runtime.InteropServices;
namespace MyLibrary
{
public class MyClass
{
[DllImport("MyDll.dll")]
public static extern int MyFunction(int arg1, float arg2);
}
}
```
2. 编译C#代码生成dll文件,将其放在一个目录下。
3. 在Python中使用ctypes库加载dll文件,并获取要调用的函数:
```python
import ctypes
# 加载dll文件
mydll = ctypes.cdll.LoadLibrary("path/to/MyDll.dll")
# 获取要调用的函数
myfunction = mydll.MyFunction
myfunction.argtypes = [ctypes.c_int, ctypes.c_float]
myfunction.restype = ctypes.c_int
```
注意,这里需要使用ctypes的LoadLibrary函数加载dll文件,并使用函数的argtypes和restype属性指定参数类型和返回值类型。
4. 将获取到的函数封装为Python函数:
```python
def my_function(arg1, arg2):
return myfunction(arg1, arg2)
```
现在就可以使用Python调用C#生成的dll文件,并调用其中的函数了。
阅读全文
相关推荐
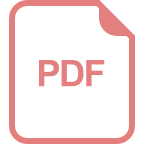


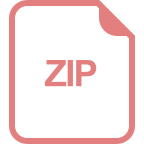
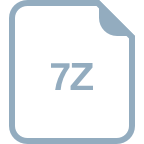
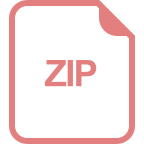
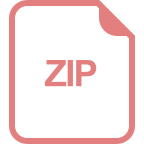
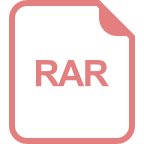
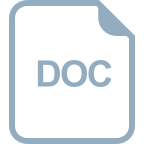
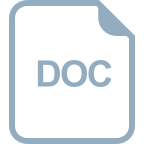
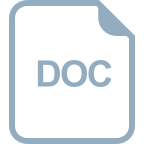
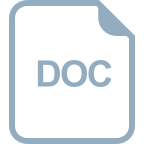
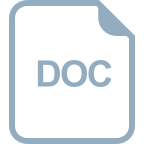



