完整代码设计实现一个@After标注的Advice,定义一个@Pointcut切点,对使用了@MyLogAnnotation标注的方法进行前置增强,并通过ApplicationContext验证它
时间: 2024-02-03 15:15:24 浏览: 84
以下是一个完整的代码示例,演示如何使用Spring AOP实现@AfterAdvice和@Pointcut:
首先,我们定义一个自定义注解@MyLogAnnotation,用于标记需要进行日志记录的方法:
```java
import java.lang.annotation.*;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface MyLogAnnotation {
}
```
然后,我们定义一个切面类,其中包含一个@Pointcut切点和一个@AfterAdvice,用于在使用了@MyLogAnnotation注解的方法执行后输出日志信息:
```java
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class MyLogAspect {
@Pointcut("@annotation(com.example.demo.MyLogAnnotation)")
public void logPointcut() {
}
@After("logPointcut()")
public void logAfter(JoinPoint joinPoint) {
System.out.println("Method " + joinPoint.getSignature().getName() + " is finished");
}
}
```
在上面的代码中,我们使用@Pointcut注解定义了一个切点logPointcut(),它匹配所有使用了@MyLogAnnotation注解的方法。然后,我们使用@After注解定义了一个增强方法logAfter(),它会在匹配的方法执行后输出一条日志信息。
最后,我们需要在Spring配置文件中将切面类配置为一个bean,以便Spring能够管理它并将其应用于目标对象。例如,在applicationContext.xml中添加以下内容:
```xml
<bean id="myLogAspect" class="com.example.demo.MyLogAspect"/>
```
现在,我们可以在任何使用了@MyLogAnnotation注解的方法上测试这个切面:
```java
import org.springframework.stereotype.Service;
@Service
public class MyService {
@MyLogAnnotation
public void doSomething() {
System.out.println("Doing something...");
}
}
```
在上面的代码中,我们使用了@MyLogAnnotation注解标记了一个方法doSomething(),这将导致切面类的logAfter()方法在该方法执行后自动被调用。
最后,我们可以使用ApplicationContext来测试这个切面是否正常工作。例如,在一个测试类中添加以下代码:
```java
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:applicationContext.xml"})
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testDoSomething() {
myService.doSomething();
}
}
```
在上面的代码中,我们使用SpringJUnit4ClassRunner来运行测试,并将applicationContext.xml配置文件加载到测试环境中。然后,我们使用@Autowired注解将MyService注入到测试类中,并在测试方法中调用doSomething()方法。如果一切正常,我们应该在控制台上看到一条日志信息,表明切面已经正常工作。
阅读全文
相关推荐
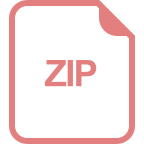
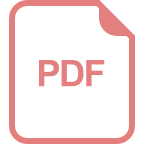
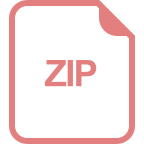


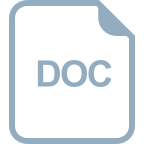
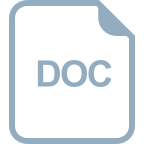
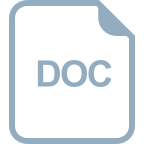
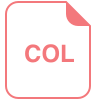
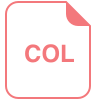
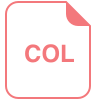
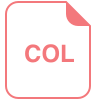
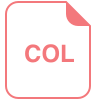
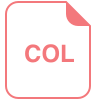
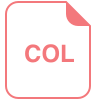
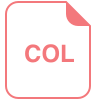
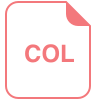
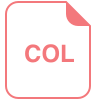
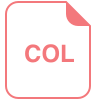
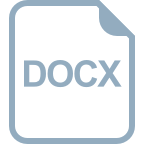