帮我写一串网页数据爬取的代码
时间: 2023-10-06 22:09:36 浏览: 68
好的,以下是一个基于Python的简单网页数据爬取示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 获取网页中的所有链接
links = []
for link in soup.find_all('a'):
links.append(link.get('href'))
# 获取网页中的所有文本
texts = []
for text in soup.find_all('p'):
texts.append(text.get_text())
# 输出结果
print(links)
print(texts)
```
这个示例使用了requests库发送HTTP请求,BeautifulSoup库解析HTML内容,获取了网页中的所有链接和文本,并将结果输出到控制台。你可以根据自己的需求,对这个示例进行修改和扩展。
相关问题
帮我写一个html的爬取蔚蓝书店的网页按钮有开始爬取,保存到MongoDB,爬取数据展示,数据爬取进度展示
好的,以下是一个简单的HTML页面代码,可以实现你所需的功能:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>蔚蓝书店数据爬取</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
$('#start-btn').click(function() {
$.ajax({
url: '/start',
type: 'GET',
success: function() {
console.log('开始爬取数据');
},
error: function() {
console.log('请求失败,请检查服务器!');
}
});
});
$('#show-btn').click(function() {
$.ajax({
url: '/show',
type: 'GET',
success: function(data) {
$('#show-data').html(data);
},
error: function() {
console.log('请求失败,请检查服务器!');
}
});
});
$('#progress-btn').click(function() {
$.ajax({
url: '/progress',
type: 'GET',
success: function(data) {
$('#progress-data').html(data);
},
error: function() {
console.log('请求失败,请检查服务器!');
}
});
});
});
</script>
</head>
<body>
<h1>蔚蓝书店数据爬取</h1>
<button id="start-btn">开始爬取</button>
<br><br>
<button id="show-btn">爬取数据展示</button>
<div id="show-data"></div>
<br><br>
<button id="progress-btn">数据爬取进度展示</button>
<div id="progress-data"></div>
</body>
</html>
```
上述代码中,我们使用了jQuery的ajax方法来实现异步请求。我们定义了三个按钮:开始爬取、爬取数据展示、数据爬取进度展示。
当点击开始爬取按钮时,发送GET请求到服务器的`/start`路由,表示开始爬取数据。当点击爬取数据展示按钮时,发送GET请求到服务器的`/show`路由,获取爬取的数据并展示在页面上。当点击数据爬取进度展示按钮时,发送GET请求到服务器的`/progress`路由,获取数据爬取的进度并展示在页面上。
接下来,我们需要在服务器端实现这些路由。
```python
from flask import Flask, render_template
from pymongo import MongoClient
import threading
import time
import requests
import json
app = Flask(__name__)
# 连接MongoDB数据库
client = MongoClient('mongodb://localhost:27017/')
db = client['bookstore']
collection = db['book']
# 定义一个全局变量,表示爬取进度
progress = 0
# 定义一个爬取函数
def spider():
global progress
# 发送GET请求获取数据
res = requests.get('http://www.bookstore.com/data.json')
# 将数据插入到MongoDB中
data = json.loads(res.text)
for book in data:
collection.insert_one(book)
progress += 1
time.sleep(0.1)
# 定义路由
@app.route('/')
def index():
return render_template('index.html')
@app.route('/start')
def start_spider():
global progress
progress = 0
# 创建一个线程,执行爬取函数
t = threading.Thread(target=spider)
t.start()
return 'OK'
@app.route('/show')
def show_data():
data = ''
# 查询MongoDB中的所有数据
for book in collection.find():
data += '书名:{},作者:{},价格:{}<br>'.format(book['name'], book['author'], book['price'])
return data
@app.route('/progress')
def show_progress():
global progress
return str(progress)
if __name__ == '__main__':
app.run(debug=True)
```
在服务器端,我们使用了Flask框架来实现路由函数。在`/start`路由函数中,我们创建了一个线程来执行爬取函数,防止爬取过程中阻塞主线程。在爬取过程中,我们将爬取进度保存在全局变量`progress`中。在`/show`路由函数中,我们查询MongoDB中的所有数据,并将其以HTML格式返回。在`/progress`路由函数中,我们直接返回全局变量`progress`的值。
注意,上述代码中的`http://www.bookstore.com/data.json`应该替换为蔚蓝书店的数据接口。另外,MongoDB的连接字符串和数据库名、集合名应该根据实际情况进行修改。
希望能对你有所帮助!
使用Java语言实现对网页的数据爬取 请写出详细代码
感谢您的提问。关于网页数据爬取,建议您先了解一些基础知识,例如HTTP协议、HTML标签等等。下面给您提供一份简单的Java代码示例,希望能够帮助您入门:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.URLConnection;
public class WebCrawler {
public static void main(String[] args) {
String url = "https://www.example.com"; // 待爬取的网页
String content = getContent(url);
System.out.println(content);
}
public static String getContent(String urlString) {
StringBuilder content = new StringBuilder();
try {
URL url = new URL(urlString);
URLConnection conn = url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
while ((inputLine = reader.readLine()) != null) {
content.append(inputLine + "\n"); // 将每行内容拼接到StringBuilder中
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
return content.toString();
}
}
```
这段代码通过URL和URLConnection类实现网络连接,通过BufferedReader读取网页内容并将其拼接到StringBuilder中,最终返回字符串形式的网页内容。当然,实际的爬虫项目可能要考虑更多细节,例如网页编码、cookies、限流等等,但这里只是一个简单示例。
阅读全文
相关推荐
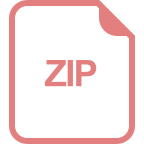
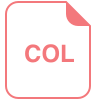
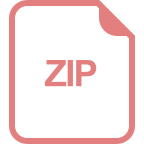
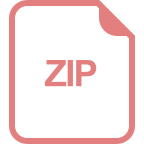
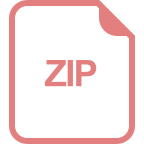
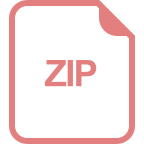
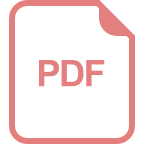
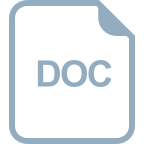






