java实现动画的代码
时间: 2023-08-06 19:13:43 浏览: 49
Java实现动画的方式有很多种,下面给出两种基本的实现方式。
1. 使用Swing的定时器(Timer)
Swing中提供了一个javax.swing.Timer类,可以方便地实现动画效果。Timer有两个构造方法:
```
Timer(int delay, ActionListener listener) // 指定延迟时间和监听器
Timer(int delay, ActionListener listener, int initialDelay) // 指定延迟时间、监听器和初始延迟时间
```
其中delay表示延迟时间,listener表示Action事件的监听器,initialDelay表示第一次延迟的时间。Timer提供了start()、stop()、setDelay()等方法来启动、停止和设置延迟时间。
下面是一个简单的使用Timer实现动画的例子:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class AnimationTest extends JPanel implements ActionListener {
private int x = 0; // 图片的x坐标
private ImageIcon imageIcon = new ImageIcon("image.jpg"); // 图片
public AnimationTest() {
Timer timer = new Timer(10, this); // 定时器,每10ms触发一次Action事件
timer.start(); // 启动定时器
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(imageIcon.getImage(), x, 0, null); // 绘制图片
}
public void actionPerformed(ActionEvent e) {
x++; // 每次移动1个像素
repaint(); // 重新绘制
}
public static void main(String[] args) {
JFrame frame = new JFrame("AnimationTest");
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new AnimationTest());
frame.setVisible(true);
}
}
```
2. 使用线程实现动画
使用线程实现动画的基本思路是在一个独立的线程中不断地更新UI界面。具体实现方式可以是:
1)在run()方法中使用while循环不断更新界面;
2)在run()方法中使用Thread.sleep()方法设置更新频率。
下面是一个使用线程实现动画的例子:
```
import javax.swing.*;
import java.awt.*;
public class AnimationTest2 extends JPanel implements Runnable {
private int x = 0; // 图片的x坐标
private ImageIcon imageIcon = new ImageIcon("image.jpg"); // 图片
public AnimationTest2() {
Thread thread = new Thread(this); // 创建线程
thread.start(); // 启动线程
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(imageIcon.getImage(), x, 0, null); // 绘制图片
}
public void run() {
while (true) {
x++; // 每次移动1个像素
repaint(); // 重新绘制
try {
Thread.sleep(10); // 线程休眠10ms
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("AnimationTest2");
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new AnimationTest2());
frame.setVisible(true);
}
}
```
以上两种实现方式都可以实现动画效果,具体选择哪种方式取决于具体应用场景和个人喜好。
相关推荐
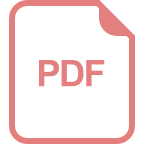
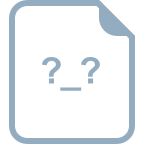














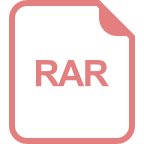