用python写一个扫雷游戏
时间: 2023-12-02 14:02:18 浏览: 35
好的,下面是一个基于 Python 的简单扫雷游戏的代码示例。这个游戏使用了 Tkinter GUI 库来创建用户界面。代码中注释会说明每个函数的作用。
```
import tkinter as tk
import random
class Minesweeper:
def __init__(self, master, rows, cols, mines):
self.master = master
self.rows = rows
self.cols = cols
self.mines = mines
self.board = [[0 for i in range(cols)] for j in range(rows)]
self.flags = [[False for i in range(cols)] for j in range(rows)]
self.tiles = []
self.game_over = False
self.create_board()
def create_board(self):
for i in range(self.rows):
row = []
for j in range(self.cols):
tile = tk.Button(self.master, width=2, height=1, command=lambda row=i, col=j: self.reveal(row, col))
tile.grid(row=i, column=j)
row.append(tile)
self.tiles.append(row)
self.place_mines()
def place_mines(self):
mines = 0
while mines < self.mines:
row = random.randint(0, self.rows-1)
col = random.randint(0, self.cols-1)
if self.board[row][col] != -1:
self.board[row][col] = -1
mines += 1
self.calculate_adjacent()
def calculate_adjacent(self):
for i in range(self.rows):
for j in range(self.cols):
if self.board[i][j] == -1:
continue
count = 0
for ni in range(max(0, i-1), min(self.rows, i+2)):
for nj in range(max(0, j-1), min(self.cols, j+2)):
if self.board[ni][nj] == -1:
count += 1
self.board[i][j] = count
def reveal(self, row, col):
if self.game_over or self.flags[row][col]:
return
if self.board[row][col] == -1:
self.game_over = True
for i in range(self.rows):
for j in range(self.cols):
if self.board[i][j] == -1:
self.tiles[i][j].config(text="*", bg="red")
return
self.tiles[row][col].config(text=self.board[row][col], bg="light gray")
if self.board[row][col] == 0:
for ni in range(max(0, row-1), min(self.rows, row+2)):
for nj in range(max(0, col-1), min(self.cols, col+2)):
if ni == row and nj == col:
continue
if self.tiles[ni][nj]["text"] == "":
self.reveal(ni, nj)
def flag(self, row, col):
if self.game_over:
return
if self.flags[row][col]:
self.flags[row][col] = False
self.tiles[row][col].config(text="")
else:
self.flags[row][col] = True
self.tiles[row][col].config(text="F")
root = tk.Tk()
root.title("Minesweeper")
game = Minesweeper(root, 10, 10, 10)
root.mainloop()
```
这个代码创建了一个 10x10 的扫雷游戏,并且包含 10 个地雷。你可以按需修改 `rows`,`cols` 和 `mines` 参数来调整游戏的规模。运行这个代码,你会看到一个简单的游戏界面。左键点击一个方格来揭开它,右键点击一个方格来标记它。
相关推荐
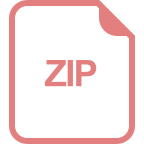


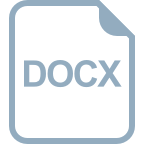
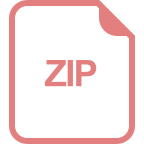
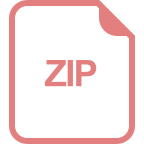
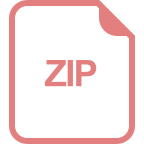
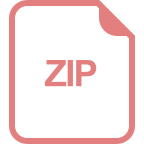
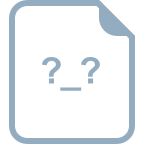
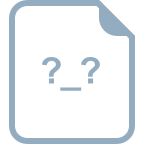