使用nodejs做一个视频播放流,可以拖动进度,要求在windows下运行,视频路径为绝对路径
时间: 2024-03-24 16:35:39 浏览: 178
在Windows下,可以使用Node.js的`child_process`模块来调用系统命令来打开视频文件。同时,为了支持绝对路径,可以使用Node.js的`path`模块来处理路径字符串。
下面是一个简单的Node.js代码示例,可以实现视频播放流和拖动进度条的功能,并在Windows系统下打开视频文件:
```javascript
const http = require('http');
const fs = require('fs');
const path = require('path');
const { exec } = require('child_process');
const videoPath = 'C:\\path\\to\\video.mp4';
http.createServer((req, res) => {
// 读取视频文件
const stat = fs.statSync(videoPath);
const fileSize = stat.size;
const range = req.headers.range;
// 计算视频范围
let start = 0;
let end = fileSize - 1;
if (range) {
const parts = range.replace(/bytes=/, '').split('-');
const partialStart = parts[0];
const partialEnd = parts[1];
start = parseInt(partialStart, 10);
end = partialEnd ? parseInt(partialEnd, 10) : fileSize - 1;
}
const chunksize = end - start + 1;
const file = fs.createReadStream(videoPath, { start, end });
const head = {
'Content-Range': `bytes ${start}-${end}/${fileSize}`,
'Accept-Ranges': 'bytes',
'Content-Length': chunksize,
'Content-Type': 'video/mp4',
};
res.writeHead(206, head);
// 把视频流传输到响应中
file.pipe(res);
}).listen(8000, () => {
console.log('Server is running on port 8000');
// 在浏览器中打开视频文件
const videoUrl = `http://localhost:8000/${path.basename(videoPath)}`;
const command = `start "" "${videoUrl}"`;
exec(command, (error) => {
if (error) {
console.error(`打开视频文件失败: ${error}`);
} else {
console.log(`正在打开视频文件: ${videoPath}`);
}
});
});
```
在上面的代码中,除了读取视频文件和设置响应头的代码与前面的示例相同外,新增了使用`child_process`模块调用系统命令的代码。其中,`path.basename()`方法用于获取视频文件的文件名,`exec()`方法用于执行系统命令。
在客户端页面中的JavaScript代码与前面的示例相同,不再赘述。
需要注意的是,由于Windows下的路径分隔符为`\`,而在JavaScript中`\`是转义字符,所以在定义路径字符串时,需要使用两个反斜杠`\\`来表示一个反斜杠。例如,视频路径为`C:\path\to\video.mp4`,则需要定义为`C:\\path\\to\\video.mp4`。
阅读全文
相关推荐
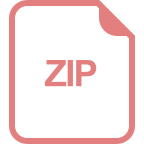
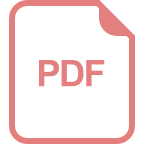
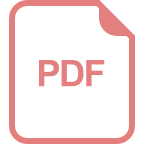
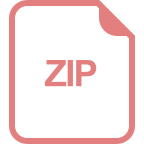
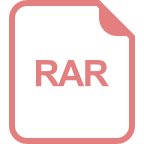
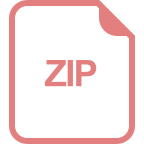
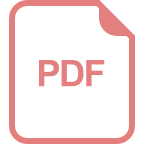
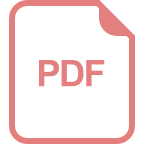
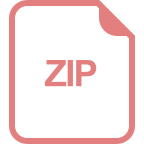
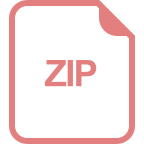
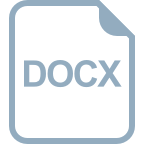
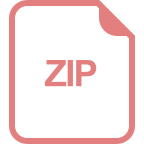
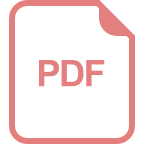
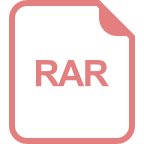