货币加减(运算符重载)
时间: 2023-08-10 08:04:13 浏览: 50
货币加减可以通过运算符重载来实现。在C++中,可以通过重载"+"和"-"运算符来实现货币加减操作。具体实现方法如下:
首先定义一个货币类Money,其中包括货币金额amount和货币单位currency。然后通过重载"+"和"-"运算符实现货币加减操作。具体代码如下:
```c++
#include <iostream>
using namespace std;
class Money {
private:
int amount;
string currency;
public:
Money(int a, string c) : amount(a), currency(c) {}
Money operator+(const Money& m) {
if (this->currency != m.currency) {
cout << "Error: currencies don't match!" << endl;
return Money(0, "");
}
return Money(this->amount + m.amount, this->currency);
}
Money operator-(const Money& m) {
if (this->currency != m.currency) {
cout << "Error: currencies don't match!" << endl;
return Money(0, "");
}
return Money(this->amount - m.amount, this->currency);
}
void print() {
cout << this->amount << " " << this->currency << endl;
}
};
int main() {
Money m1(100, "USD");
Money m2(200, "USD");
Money m3(50, "EUR");
Money sum = m1 + m2;
Money diff = m2 - m1;
Money error = m1 + m3;
sum.print(); // 输出300 USD
diff.print(); // 输出100 USD
error.print(); // 输出错误信息
return 0;
}
```
在上面的代码中,重载的"+"和"-"运算符都返回一个Money对象,其中包括两个货币对象的金额之和或差以及货币单位。在进行加减运算时,需要先判断两个货币对象的单位是否相同,如果不同则输出错误信息并返回一个空的Money对象。
注意,在实际应用中,货币加减运算可能涉及到货币汇率的转换等问题,需要进行更为复杂的处理。
相关推荐
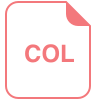
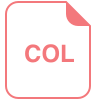
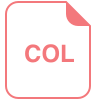
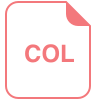
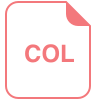









