python获取value为特定值的key
时间: 2024-05-14 12:16:30 浏览: 8
可以使用字典推导式来实现,例如:
```python
my_dict = {'apple': 1, 'banana': 2, 'orange': 1, 'peach': 3}
target_value = 1
result = [key for key, value in my_dict.items() if value == target_value]
print(result) # 输出 ['apple', 'orange']
```
上面的代码中,首先定义了一个字典 `my_dict`,然后指定了目标值 `target_value` 为 1。接下来使用字典推导式来遍历字典中的每个键值对,只有当值等于目标值时,才将对应的键添加到结果列表中。最后打印结果列表即可。
相关问题
如何使用python从响应中获取特定值
可以使用Python中的requests库发送HTTP请求,然后使用json库解析响应中的JSON格式数据。通过键-值对的形式,可以方便地从JSON中获取特定的值。例如:
```
import requests
import json
url = 'https://api.example.com/get_info'
response = requests.get(url)
data = json.loads(response.text)
specific_value = data['key']
```
其中,`get_info`是API的地址,`key`是我们要获取的特定值的键名。可以根据具体的API文档来确定地址和键名。
python获取dict中某个key其值出现次数
可以使用Python中的collections模块中的Counter类来实现。
例如,假设我们有一个字典my_dict,它包含多个key和value。现在,我们想要统计其中一个特定key的出现次数,可以使用以下代码:
```
from collections import Counter
my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 1, 'e': 2, 'f': 1}
key_to_count = 'a'
counts = Counter([value for key, value in my_dict.items() if key == key_to_count])
print(counts[key_to_count])
```
在上面的代码中,我们首先从collections模块中导入Counter类。然后,我们定义了一个my_dict字典,其中包含多个key和value。
接下来,我们定义了一个key_to_count变量,它包含我们想要统计的key的名称。然后,我们使用列表推导式从my_dict中获取所有与key_to_count相同的值,并将它们传递给Counter类。
最后,我们打印出Counter对象中key_to_count对应的值的出现次数。
输出结果为:
```
3
```
这意味着在my_dict字典中,key为'a'的值出现了3次。
相关推荐
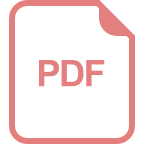
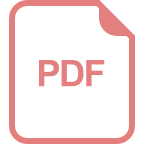
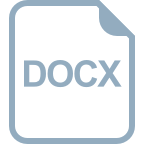
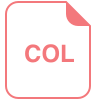











