使用pycharm对图像进行处理提取轮廓,测量尺寸
时间: 2023-11-17 10:53:42 浏览: 112
以下是使用Pycharm对图像进行处理提取轮廓,测量尺寸的步骤:
1. 导入相关的库和模块
```python
import cv2
import numpy as np
```
2. 加载图像
```python
img = cv2.imread('image.jpg')
```
3. 灰度化处理
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
4. 滤波处理
```python
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
```
5. 边缘检测
```python
edged = cv2.Canny(blurred, 30, 150)
```
6. 轮廓提取
```python
contours, hierarchy = cv2.findContours(edged, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
7. 绘制轮廓
```python
cv2.drawContours(img, contours, -1, (0, 0, 255), 2)
```
8. 测量尺寸
```python
for c in contours:
# 计算轮廓周长
perimeter = cv2.arcLength(c, True)
# 计算轮廓面积
area = cv2.contourArea(c)
# 计算轮廓包围框
x, y, w, h = cv2.boundingRect(c)
# 绘制包围框
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示测量结果
print("Perimeter: {:.2f}".format(perimeter))
print("Area: {:.2f}".format(area))
```
9. 显示处理结果
```python
cv2.imshow("Contours", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
edged = cv2.Canny(blurred, 30, 150)
contours, hierarchy = cv2.findContours(edged, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0, 0, 255), 2)
for c in contours:
perimeter = cv2.arcLength(c, True)
area = cv2.contourArea(c)
x, y, w, h = cv2.boundingRect(c)
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
print("Perimeter: {:.2f}".format(perimeter))
print("Area: {:.2f}".format(area))
cv2.imshow("Contours", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
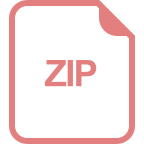