两种方式(手动实现、sklearn实现)构建圣保罗市房价数据的线性回归模型。 2.在手动实现的过程中,尝试: ①用图的形式来展示迭代过程中假设函数的变化。 ②设定一个阈值,当参数变化小于阈值时,令梯度下降算法停止。并用jupyter notebook写出代码
时间: 2023-03-27 11:00:42 浏览: 248
对于您的第一个问题,我可以回答。对于圣保罗市房价数据的线性回归模型,可以采用手动实现和sklearn实现两种方式。手动实现需要先对数据进行预处理,包括数据清洗、特征选择和数据标准化等步骤,然后使用梯度下降算法来求解模型参数。而sklearn实现则可以直接调用库函数来完成模型的训练和预测。
对于您的第二个问题,如果采用手动实现的方式,可以尝试用图的形式来展示迭代过程中假设函数的变化,这有助于理解梯度下降算法的工作原理。同时,可以设定一个阈值,当参数变化小于阈值时,令梯度下降算法停止,这可以提高算法的收敛速度和准确性。以下是手动实现的代码示例:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 加载数据
data = pd.read_csv('sao-paulo-real-estate-sale-rent-april-2019.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 特征标准化
mu = np.mean(X, axis=)
sigma = np.std(X, axis=)
X = (X - mu) / sigma
# 添加偏置项
X = np.hstack((np.ones((X.shape[], 1)), X))
# 初始化参数
theta = np.zeros(X.shape[1])
# 定义代价函数
def cost_function(X, y, theta):
m = len(y)
J = np.sum((X.dot(theta) - y) ** 2) / (2 * m)
return J
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, threshold):
m = len(y)
J_history = [cost_function(X, y, theta)]
while True:
delta = X.T.dot(X.dot(theta) - y) / m
theta -= alpha * delta
J_history.append(cost_function(X, y, theta))
if abs(J_history[-1] - J_history[-2]) < threshold:
break
return theta, J_history
# 训练模型
alpha = .01
threshold = 1e-5
theta, J_history = gradient_descent(X, y, theta, alpha, threshold)
# 绘制代价函数曲线
plt.plot(J_history)
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.show()
```
阅读全文
相关推荐
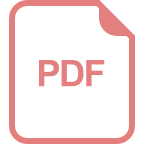

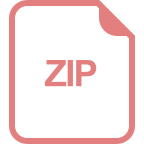
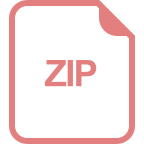
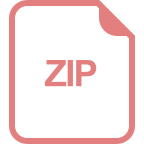
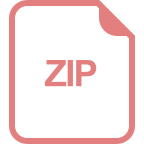
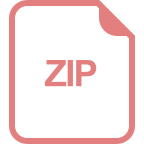
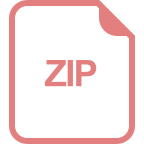
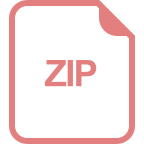
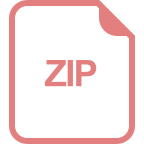
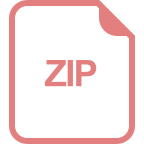
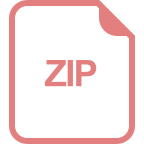
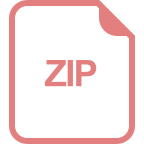
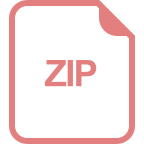
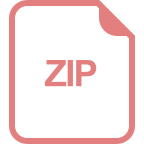