wpf购物车ui页面设计和控制器代码
时间: 2024-02-08 08:38:35 浏览: 189
以下是WPF购物车UI页面设计和控制器代码的示例:
XAML页面代码:
```xml
<Window x:Class="ShoppingCart.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Shopping Cart" Height="450" Width="800">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="250" />
</Grid.ColumnDefinitions>
<ListView x:Name="ProductsListView" Grid.Column="0" Margin="10">
<ListView.View>
<GridView>
<GridViewColumn Header="Product Name" DisplayMemberBinding="{Binding Name}" Width="200"/>
<GridViewColumn Header="Price" DisplayMemberBinding="{Binding Price}" Width="100"/>
</GridView>
</ListView.View>
</ListView>
<Grid Grid.Column="1" Margin="10">
<Grid.RowDefinitions>
<RowDefinition Height="40" />
<RowDefinition Height="*" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<TextBlock Text="Shopping Cart" FontSize="20" FontWeight="Bold" Margin="0,0,0,10" />
<ListBox x:Name="CartListBox" Grid.Row="1">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" Margin="0,0,10,0" />
<TextBlock Text="{Binding Price, StringFormat='{}${0:N2}'}" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<Button Content="Checkout" Grid.Row="2" Width="100" Margin="0,10,0,0" Click="CheckoutButton_Click" />
<TextBlock x:Name="TotalTextBlock" Grid.Row="3" Margin="0,10,0,0" />
</Grid>
</Grid>
</Window>
```
控制器代码:
```csharp
public partial class MainWindow : Window
{
private List<Product> products = new List<Product>();
private List<Product> cart = new List<Product>();
public MainWindow()
{
InitializeComponent();
// 添加商品数据
products.Add(new Product("Product 1", 10.99));
products.Add(new Product("Product 2", 19.99));
products.Add(new Product("Product 3", 7.50));
products.Add(new Product("Product 4", 25.00));
// 绑定商品数据到 ListView
ProductsListView.ItemsSource = products;
}
private void CheckoutButton_Click(object sender, RoutedEventArgs e)
{
// 计算购物车总价
double total = cart.Sum(p => p.Price);
// 显示总价
TotalTextBlock.Text = $"Total: ${total:N2}";
// 清空购物车
cart.Clear();
CartListBox.ItemsSource = null;
}
private void ProductsListView_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
// 将选中的商品添加到购物车
if (ProductsListView.SelectedItem != null)
{
Product selectedProduct = (Product)ProductsListView.SelectedItem;
cart.Add(selectedProduct);
CartListBox.ItemsSource = cart;
}
}
}
public class Product
{
public string Name { get; set; }
public double Price { get; set; }
public Product(string name, double price)
{
Name = name;
Price = price;
}
}
```
该示例创建了一个简单的购物车UI,包含了两个列表控件:一个显示商品列表,另一个显示购物车列表。当用户选中一个商品时,该商品将被添加到购物车中。当用户点击结算按钮时,购物车中所有商品的总价将被计算并显示在UI上。购物车将被清空以便下一次使用。
阅读全文
相关推荐
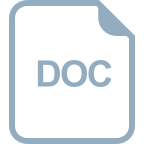
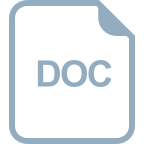
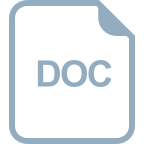

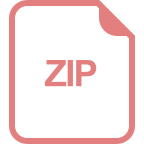
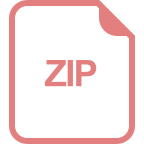
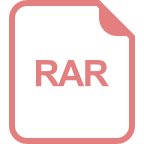
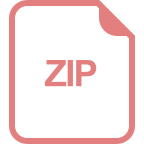
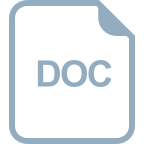
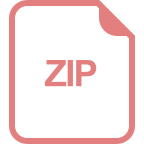
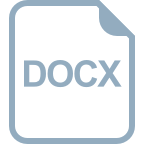
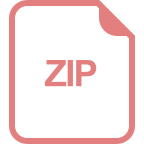
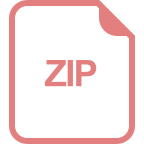
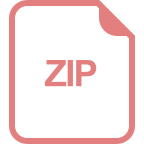
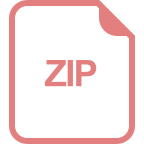
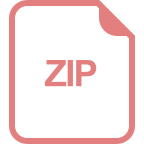