python用gui设计背单词程序
时间: 2024-05-09 13:16:02 浏览: 206
可以使用Python自带的Tkinter模块来设计GUI界面,再结合一个背单词的数据文件,实现背单词程序的功能。
以下是一个简单的例子,展示如何使用Tkinter创建一个窗口,包含一个显示单词的标签和一个按钮,点击按钮可以显示下一个单词。
```python
import tkinter as tk
class Vocabulary:
def __init__(self):
# 读取单词数据文件
self.words = []
with open('words.txt', 'r') as f:
for line in f:
word, meaning = line.strip().split(':')
self.words.append((word, meaning))
# 当前单词索引
self.index = 0
def next_word(self):
# 显示下一个单词
self.index = (self.index + 1) % len(self.words)
word, meaning = self.words[self.index]
self.word_label.config(text=word)
self.meaning_label.config(text='')
def show_meaning(self):
# 显示单词的意思
_, meaning = self.words[self.index]
self.meaning_label.config(text=meaning)
# 创建窗口
root = tk.Tk()
root.title('背单词程序')
# 创建单词和意思标签
vocab = Vocabulary()
word_label = tk.Label(root, text=vocab.words[0][0], font=('Arial', 36))
word_label.pack()
meaning_label = tk.Label(root, font=('Arial', 24))
meaning_label.pack()
# 创建下一个单词按钮
next_button = tk.Button(root, text='下一个', command=vocab.next_word)
next_button.pack()
# 创建显示意思按钮
meaning_button = tk.Button(root, text='显示意思', command=vocab.show_meaning)
meaning_button.pack()
# 进入主循环
root.mainloop()
```
需要准备一个单词数据文件`words.txt`,每行一个单词和它的意思,用冒号隔开。例如:
```
apple:苹果
banana:香蕉
orange:橙子
```
当单击“下一个”按钮时,程序会显示下一个单词;当单击“显示意思”按钮时,程序会显示当前单词的意思。
这只是一个简单的例子,您可以根据自己的需求进行更改和扩展。
阅读全文
相关推荐
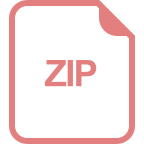
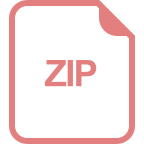
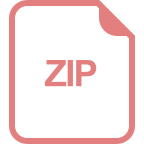
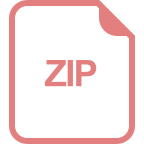
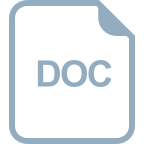

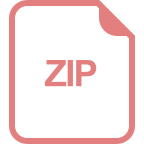
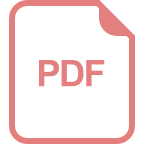
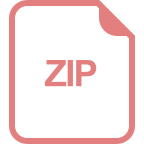
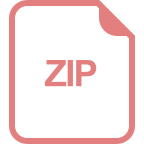
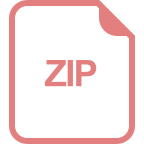
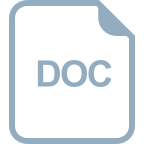
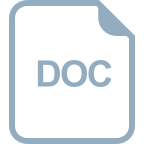
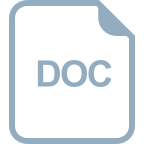
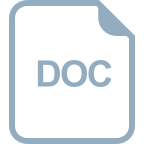

