python 如何修改 mysql 的一条数据
时间: 2024-03-21 16:38:33 浏览: 19
要修改 MySQL 数据库中的一条数据,你可以使用 UPDATE 语句。下面是一个示例代码,可以修改一个名为 "users" 的表中的数据:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
mycursor = mydb.cursor()
sql = "UPDATE users SET name = 'John' WHERE id = 1"
mycursor.execute(sql)
mydb.commit()
print(mycursor.rowcount, "record(s) updated")
```
这个示例代码将名为 "users" 的表中 id 为 1 的记录的名称修改为 "John"。注意在执行 UPDATE 语句后要使用 commit() 函数提交修改,否则修改不会生效。
相关问题
python mysql 插入一条数据的过程代码
好的,Python 中插入一条数据到 MySQL 数据库的过程一般如下:
1. 连接 MySQL 数据库
使用 Python 中的 `mysql.connector` 模块连接 MySQL 数据库,示例代码如下:
```
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="database_name"
)
```
其中,`host` 是 MySQL 服务器地址,`user` 和 `password` 是登录 MySQL 的用户名和密码,`database` 是要连接的数据库名称。
2. 创建游标对象
使用 `cursor()` 方法创建游标对象,该对象用于执行 SQL 语句,示例代码如下:
```
mycursor = mydb.cursor()
```
3. 编写 SQL 语句
编写要执行的 SQL 语句,示例代码如下:
```
sql = "INSERT INTO student (id, name, age) VALUES (%s, %s, %s)"
val = (1, "Tom", 18)
```
其中,`sql` 是要执行的 SQL 语句,`VALUES` 后面的 `%s` 是占位符,`val` 是要插入的具体数值,这里用了元组的形式。
4. 执行 SQL 语句
使用 `execute()` 方法执行 SQL 语句,示例代码如下:
```
mycursor.execute(sql, val)
mydb.commit()
```
其中,`execute()` 方法的第一个参数是要执行的 SQL 语句,第二个参数是要插入的具体数值。`commit()` 方法用于提交事务,保存更改。
5. 关闭游标和数据库连接
执行完 SQL 语句后,需要关闭游标和数据库连接,示例代码如下:
```
mycursor.close()
mydb.close()
```
这样就完成了 Python 中插入一条数据到 MySQL 数据库的过程。
python 如何向mysql 快速插入一条数据
Python 可以使用 MySQL Connector 模块来连接 MySQL 数据库,并使用 execute() 方法向 MySQL 数据库中插入一条数据。
以下是示例代码,假设我们要向 MySQL 数据库中的 "test_table" 表中插入一条记录:
```python
import mysql.connector
# 连接 MySQL 数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 获取游标
mycursor = mydb.cursor()
# 插入记录
sql = "INSERT INTO test_table (name, age) VALUES (%s, %s)"
val = ("John", "30")
mycursor.execute(sql, val)
# 提交事务
mydb.commit()
# 输出插入的记录 ID
print("Record inserted successfully. ID:", mycursor.lastrowid)
```
在这个示例中,我们首先创建了一个 MySQL 数据库连接,然后获取了一个游标对象。接着,我们使用 execute() 方法插入了一条记录,并使用 commit() 方法提交了这个事务。最后,我们输出了插入的记录 ID。
需要注意的是,为了防止 SQL 注入攻击,我们使用了参数化查询,并将插入的值作为参数传递给 execute() 方法。这样可以确保插入的数据是安全的。
相关推荐
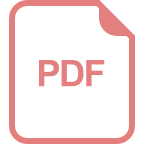
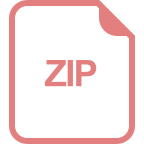
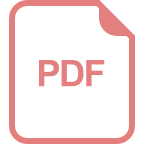












