用java编写出gui图形界面实现反复随机生成字符让用户输入,最后统计时间和正确率
时间: 2024-05-12 13:15:42 浏览: 80
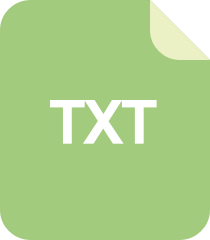
C++,C#,Java,Python实现简单的图形用户界面的区别和方法

以下是一个简单的示例代码,实现了生成随机字符并记录时间和正确率,采用Java Swing实现GUI界面:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class RandomCharGame extends JFrame implements ActionListener {
private static final int WIDTH = 400;
private static final int HEIGHT = 200;
private static final int TOTAL_TIME = 30;
private static final String CHAR_SOURCE = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
private static final int CHAR_LENGTH = CHAR_SOURCE.length();
private static final int CHAR_SIZE = 50;
private JTextField inputField;
private JLabel charLabel;
private JLabel timeLabel;
private JLabel correctLabel;
private JButton startButton;
private JButton restartButton;
private String currentChar;
private int correctCount;
private int totalCount;
private int timeLeft;
private boolean isRunning;
public RandomCharGame() {
setTitle("Random Char Game");
setSize(WIDTH, HEIGHT);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
inputField = new JTextField();
inputField.setPreferredSize(new Dimension(CHAR_SIZE, CHAR_SIZE));
inputField.addActionListener(this);
charLabel = new JLabel();
charLabel.setFont(new Font("Arial", Font.BOLD, CHAR_SIZE));
timeLabel = new JLabel();
timeLabel.setFont(new Font("Arial", Font.PLAIN, CHAR_SIZE));
timeLabel.setHorizontalAlignment(SwingConstants.RIGHT);
correctLabel = new JLabel();
correctLabel.setFont(new Font("Arial", Font.PLAIN, CHAR_SIZE));
correctLabel.setHorizontalAlignment(SwingConstants.RIGHT);
startButton = new JButton("Start");
startButton.addActionListener(this);
restartButton = new JButton("Restart");
restartButton.addActionListener(this);
restartButton.setEnabled(false);
JPanel panel = new JPanel(new GridLayout(3, 2, 10, 10));
panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
panel.add(new JLabel("Input:"));
panel.add(inputField);
panel.add(new JLabel("Char:"));
panel.add(charLabel);
panel.add(new JLabel("Time:"));
panel.add(timeLabel);
panel.add(new JLabel("Correct:"));
panel.add(correctLabel);
panel.add(startButton);
panel.add(restartButton);
getContentPane().add(panel);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
startGame();
} else if (e.getSource() == restartButton) {
restartGame();
} else if (e.getSource() == inputField) {
checkInput();
}
}
private void startGame() {
correctCount = 0;
totalCount = 0;
timeLeft = TOTAL_TIME;
isRunning = true;
startButton.setEnabled(false);
restartButton.setEnabled(false);
inputField.setEnabled(true);
inputField.setText("");
updateChar();
updateTime();
updateCorrect();
inputField.requestFocus();
new Thread(() -> {
while (timeLeft >= 0) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
timeLeft--;
updateTime();
}
isRunning = false;
inputField.setEnabled(false);
startButton.setEnabled(false);
restartButton.setEnabled(true);
}).start();
}
private void restartGame() {
startButton.setEnabled(true);
restartButton.setEnabled(false);
charLabel.setText("");
timeLabel.setText("");
correctLabel.setText("");
}
private void checkInput() {
if (!isRunning) {
return;
}
String input = inputField.getText().trim();
if (input.equals(currentChar)) {
correctCount++;
}
totalCount++;
updateChar();
updateCorrect();
inputField.setText("");
inputField.requestFocus();
}
private void updateChar() {
int index = (int) (Math.random() * CHAR_LENGTH);
currentChar = CHAR_SOURCE.substring(index, index + 1);
charLabel.setText(currentChar);
}
private void updateTime() {
timeLabel.setText(String.format(" %02d s", timeLeft));
}
private void updateCorrect() {
correctLabel.setText(String.format("%d/%d", correctCount, totalCount));
}
public static void main(String[] args) {
new RandomCharGame();
}
}
```
在这个例子中,我们使用了Java Swing库来创建GUI界面。在GUI界面中,我们有一个文本框用于用户输入,一个标签用于显示随机字符,一个标签用于显示剩余时间,一个标签用于显示正确率,以及两个按钮,一个用于开始游戏,一个用于重新开始游戏。
我们使用一个字符串来存储可选字符集,每次生成随机字符时,我们从这个字符串中随机选择一个字符。我们使用一个计时器来记录游戏时间,每秒钟更新一次剩余时间。在用户输入时,我们检查输入是否与当前字符相同,如果相同则增加正确计数器,总计数器也会增加。
最后,我们使用线程来处理时间计时器,防止主线程被阻塞。每个按钮和文本框都具有ActionListener,以便在用户单击按钮或按下回车键时触发相应的操作。
阅读全文
相关推荐
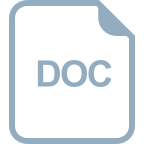
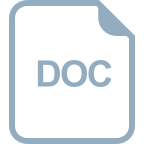
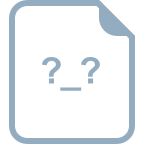
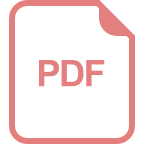
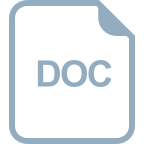
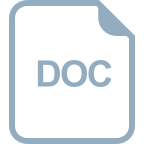
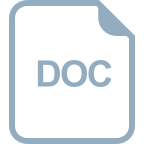
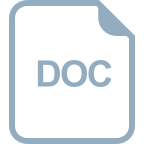
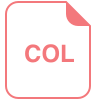
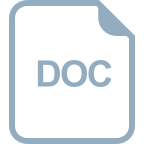
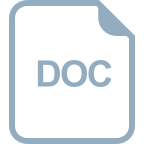
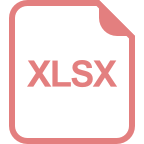
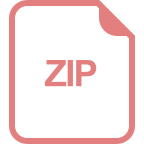
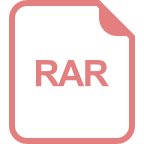
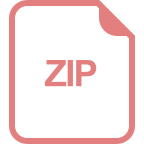
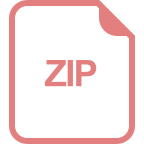
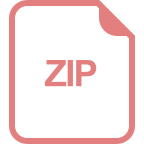
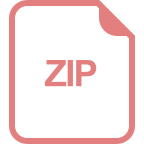
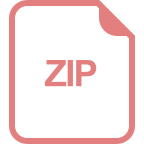