Vue项目通过scrollIntoView实现锚点点击页面平滑滚动
时间: 2023-06-02 21:01:38 浏览: 195
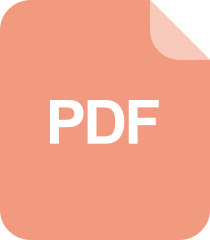
基于vue监听滚动事件实现锚点链接平滑滚动的方法
在Vue项目中,我们可以通过scrollIntoView方法实现锚点点击页面平滑滚动。
首先,在需要滚动的元素上绑定一个ref属性:
```html
<div ref="scrollElem">需要滚动的元素</div>
```
然后,在对应的锚点上绑定一个点击事件,触发滚动操作:
```html
<a @click="scrollToElem">锚点</a>
```
在Vue组件中,定义scrollToElem方法,通过ref获取需要滚动的元素,并调用scrollIntoView方法实现平滑滚动:
```js
methods: {
scrollToElem() {
this.$refs.scrollElem.scrollIntoView({
behavior: 'smooth', // 平滑滚动
block: 'start' // 滚动到元素顶部
})
}
}
```
这样,当点击锚点时,页面就会平滑滚动到对应的元素位置。
阅读全文
相关推荐
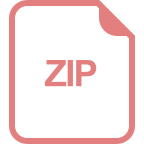
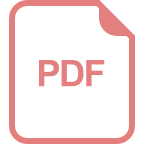

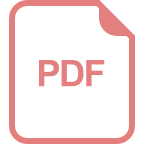
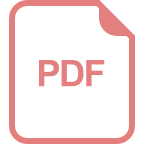
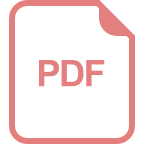
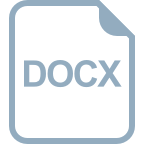









