通过线性布局和相对布局来搭建一个用户登录界面,界面可自主设计,但至少需要包括以下控件:1个ImageView控件、2个TextView控件; 2个EditText控件、 1个Button控件。 创建工具类SPSaveLogin,在该类中实现保存与获取登录账号和密码的功能,使用SharedPreferences存储的方式保存与读取登录账号与密码的数据 。运行程序,输入账号和密码信息,点击“登录”按钮,实现登录功能。
时间: 2024-03-10 21:49:44 浏览: 224
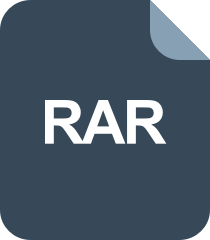
用相对布局实现登录界面设计

好的,我会尽力回答你的问题。首先,关于用户登录界面的搭建,以下是一个简单的示例代码:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/image_view"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/ic_launcher" />
<TextView
android:id="@+id/text_view_username"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/image_view"
android:text="Username:"
android:textSize="20sp" />
<EditText
android:id="@+id/edit_text_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/text_view_username"
android:hint="Enter your username" />
<TextView
android:id="@+id/text_view_password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/edit_text_username"
android:text="Password:"
android:textSize="20sp" />
<EditText
android:id="@+id/edit_text_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/text_view_password"
android:hint="Enter your password"
android:inputType="textPassword" />
<Button
android:id="@+id/button_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/edit_text_password"
android:text="Login" />
</RelativeLayout>
```
这个布局文件使用了相对布局来控制各个控件的位置。其中,ImageView用于显示logo或者其他图片;TextView用于显示提示文字;EditText用于输入用户名和密码;Button用于触发登录操作。你可以根据自己的需求进行修改和美化。
接下来,我们需要实现保存和获取登录账号和密码的功能。可以通过SharedPreferences来实现这个功能。以下是一个简单的SPSaveLogin工具类的示例代码:
```java
public class SPSaveLogin {
private SharedPreferences sharedPreferences;
private SharedPreferences.Editor editor;
public SPSaveLogin(Context context) {
sharedPreferences = context.getSharedPreferences("login", Context.MODE_PRIVATE);
editor = sharedPreferences.edit();
}
public void save(String username, String password) {
editor.putString("username", username);
editor.putString("password", password);
editor.apply();
}
public String getUsername() {
return sharedPreferences.getString("username", "");
}
public String getPassword() {
return sharedPreferences.getString("password", "");
}
}
```
在这个示例代码中,我们通过SharedPreferences来保存和获取登录账号和密码。首先,我们在构造函数中获取SharedPreferences对象和SharedPreferences.Editor对象。然后,我们提供了save()方法用于保存登录账号和密码,getUsername()和getPassword()方法用于获取登录账号和密码。
最后,我们需要在登录按钮的点击事件中调用SPSaveLogin类来实现登录功能。以下是一个简单的示例代码:
```java
public class LoginActivity extends AppCompatActivity {
private EditText mEditTextUsername;
private EditText mEditTextPassword;
private Button mButtonLogin;
private SPSaveLogin mSPSaveLogin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mEditTextUsername = findViewById(R.id.edit_text_username);
mEditTextPassword = findViewById(R.id.edit_text_password);
mButtonLogin = findViewById(R.id.button_login);
mSPSaveLogin = new SPSaveLogin(this);
mButtonLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String username = mEditTextUsername.getText().toString();
String password = mEditTextPassword.getText().toString();
mSPSaveLogin.save(username, password);
// 在这里实现登录功能
}
});
}
}
```
在这个示例代码中,我们在登录按钮的点击事件中获取用户输入的登录账号和密码,然后调用SPSaveLogin类的save()方法来保存登录账号和密码。接下来,我们可以在save()方法的后面实现登录功能,例如打开主界面或者显示登录成功的提示信息。
希望这个示例代码对你有所帮助!
阅读全文
相关推荐

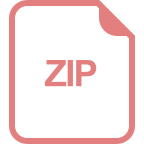
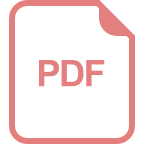
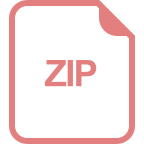
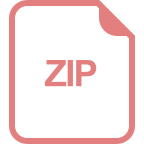
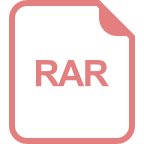
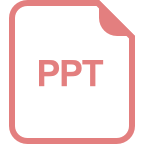
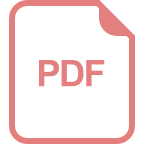
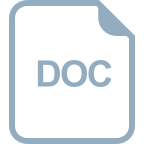
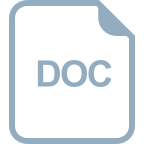
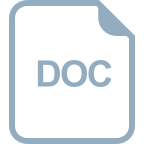
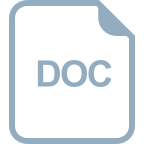
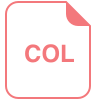
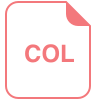
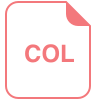
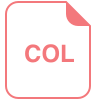
