Next, define a Dog class that is derived from Pet. The Dog class should have a private member variable named breed that stores the breed of the dog. Add mutator and accessor functions for the breed variable and appropriate constructors. Redefine the getLifespan function to return "Approximately 7 years" if the dog’s weight is over 100 pounds and "Approximately 13 years" if the dog’s weight is under 100 pounds.
时间: 2024-03-24 15:39:53 浏览: 16
Sure, here's a possible implementation of the Dog class in Python, derived from the previous Pet class:
```python
class Dog(Pet):
def __init__(self, name, age, weight, breed):
super().__init__(name, age, weight)
self.__breed = breed
def get_breed(self):
return self.__breed
def set_breed(self, breed):
self.__breed = breed
def get_lifespan(self):
if self.get_weight() > 100:
return "Approximately 7 years"
else:
return "Approximately 13 years"
```
The Dog class inherits from the Pet class using the `super()` function, and adds a private attribute `__breed` to store the breed of the dog. Note that the double underscore prefix makes the attribute private, meaning that it can only be accessed from within the class using the accessor and mutator methods.
The `get_breed` and `set_breed` methods are the accessor and mutator functions for the breed attribute.
The `get_lifespan` method is redefined to return "Approximately 7 years" if the dog's weight is over 100 pounds, and "Approximately 13 years" otherwise, as requested.
Here's an example of how to use the Dog class:
```python
# create a new Dog object
my_dog = Dog("Rufus", 5, 120, "Bulldog")
# get the name, age, weight, and breed of the dog
print(my_dog.get_name()) # output: Rufus
print(my_dog.get_age()) # output: 5
print(my_dog.get_weight()) # output: 120
print(my_dog.get_breed()) # output: Bulldog
# set a new weight and breed for the dog
my_dog.set_weight(90)
my_dog.set_breed("Golden Retriever")
print(my_dog.get_weight()) # output: 90
print(my_dog.get_breed()) # output: Golden Retriever
# get the lifespan of the dog
print(my_dog.get_lifespan()) # output: Approximately 13 years
```
相关推荐
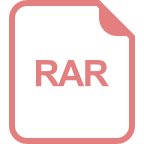
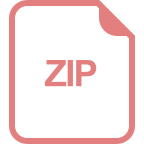
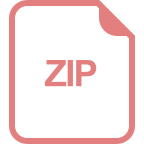
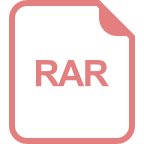
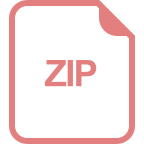
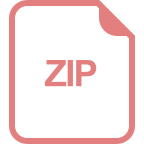
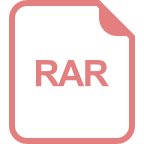
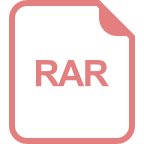
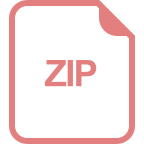
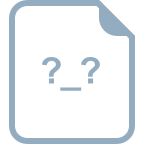
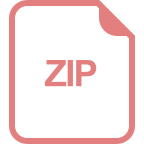
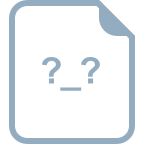
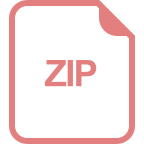
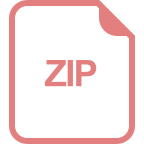