CANoe15中,使用CAPL语言实现一个tcp sever连接多个tcp client的代码
时间: 2024-05-11 11:17:29 浏览: 188
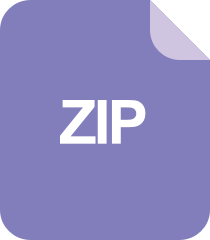
基于TCP连接的server_client连接代码

以下是一个简单的使用CAPL语言实现一个TCP服务器连接多个TCP客户端的代码示例:
```
// 定义TCP服务器和客户端的句柄
long server_handle;
long client_handle[10];
// 定义需要监听的端口号
int port = 1234;
// 定义一个TCP服务器连接回调函数
on tcpServerEvent(long handle, int event)
{
if (event == TCPS_EVENT_CONNECT) // 有客户端连接
{
for (int i = 0; i < 10; i++) // 查找空闲的客户端句柄
{
if (client_handle[i] == 0) // 找到一个空闲的句柄
{
// 接受连接并保存客户端句柄
client_handle[i] = tcpServerAccept(handle);
break;
}
}
}
}
// 在启动时创建TCP服务器并注册回调函数
on start()
{
// 创建TCP服务器
server_handle = tcpServerCreate(port);
// 注册TCP服务器连接回调函数
tcpServerSetCallback(server_handle, tcpServerEvent);
}
// 在停止时关闭所有TCP客户端和服务器
on stop()
{
// 关闭所有TCP客户端
for (int i = 0; i < 10; i++)
{
if (client_handle[i] != 0)
{
tcpClose(client_handle[i]);
client_handle[i] = 0;
}
}
// 关闭TCP服务器
tcpClose(server_handle);
}
// 在周期触发时向所有客户端发送数据
on timer()
{
char data[100];
sprintf(data, "Hello, client!\r\n");
for (int i = 0; i < 10; i++)
{
if (client_handle[i] != 0)
{
tcpSend(client_handle[i], data, strlen(data));
}
}
}
```
在这个例子中,我们首先定义了一个TCP服务器和10个TCP客户端的句柄。然后我们定义了一个TCP服务器连接回调函数,当有客户端连接时,我们会接受连接并保存客户端句柄。在启动时,我们创建TCP服务器并注册回调函数。在停止时,我们关闭所有TCP客户端和服务器。在周期触发时,我们向所有客户端发送数据。
请注意,这仅是一个简单的示例代码,您可能需要根据您的具体需求进行修改。
阅读全文
相关推荐
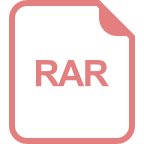
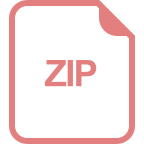
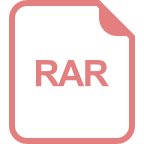
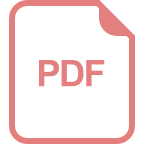
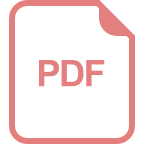
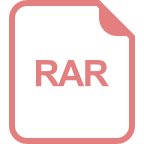
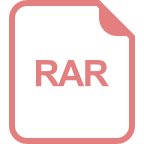
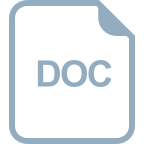
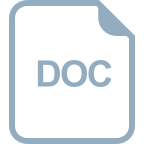
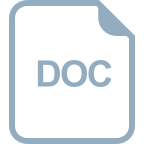
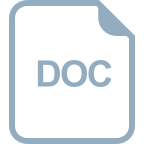




