java设计生成一个有JFrame,Text,Button,JcomboBox等组件,有相对应的鼠标事件,键盘事件和动作事件的投票小程序布局管理
时间: 2023-12-08 21:04:17 浏览: 66
好的,我可以为您提供一个简单的投票小程序的布局设计和代码实现。
首先,我们需要创建一个JFrame窗口,然后在窗口中添加所需的组件。这里我们需要使用布局管理器来布置组件。推荐使用GridBagLayout布局管理器,因为它提供了更大的灵活性和自定义性。
以下是程序的代码实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class VotingApp extends JFrame implements ActionListener, ItemListener {
private JLabel nameLabel, candidateLabel, voteLabel;
private JTextField nameField;
private JComboBox candidateBox;
private JTextArea voteArea;
private JButton voteButton;
private int candidate1Votes, candidate2Votes, candidate3Votes;
public VotingApp() {
setTitle("Voting App");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 300);
setLocationRelativeTo(null);
// Initialize components
nameLabel = new JLabel("Name:");
candidateLabel = new JLabel("Candidate:");
voteLabel = new JLabel("Votes:");
nameField = new JTextField(20);
candidateBox = new JComboBox(new String[] {"Candidate 1", "Candidate 2", "Candidate 3"});
voteArea = new JTextArea(10, 30);
voteButton = new JButton("Vote");
candidate1Votes = 0;
candidate2Votes = 0;
candidate3Votes = 0;
// Set layout manager
setLayout(new GridBagLayout());
GridBagConstraints gc = new GridBagConstraints();
gc.anchor = GridBagConstraints.WEST;
gc.insets = new Insets(5, 5, 5, 5);
// Add components to the frame
gc.gridx = 0;
gc.gridy = 0;
add(nameLabel, gc);
gc.gridx = 1;
gc.gridy = 0;
add(nameField, gc);
gc.gridx = 0;
gc.gridy = 1;
add(candidateLabel, gc);
gc.gridx = 1;
gc.gridy = 1;
add(candidateBox, gc);
gc.gridx = 0;
gc.gridy = 2;
add(voteLabel, gc);
gc.gridx = 1;
gc.gridy = 2;
add(new JScrollPane(voteArea), gc);
gc.gridx = 1;
gc.gridy = 3;
add(voteButton, gc);
// Add event listeners
candidateBox.addItemListener(this);
voteButton.addActionListener(this);
setVisible(true);
}
public void itemStateChanged(ItemEvent e) {
// Display candidate information
if (e.getStateChange() == ItemEvent.SELECTED) {
String candidate = (String) e.getItem();
if (candidate.equals("Candidate 1")) {
voteArea.setText("Candidate 1\nVotes: " + candidate1Votes);
} else if (candidate.equals("Candidate 2")) {
voteArea.setText("Candidate 2\nVotes: " + candidate2Votes);
} else if (candidate.equals("Candidate 3")) {
voteArea.setText("Candidate 3\nVotes: " + candidate3Votes);
}
}
}
public void actionPerformed(ActionEvent e) {
// Process vote
String name = nameField.getText();
String candidate = (String) candidateBox.getSelectedItem();
if (candidate.equals("Candidate 1")) {
candidate1Votes++;
} else if (candidate.equals("Candidate 2")) {
candidate2Votes++;
} else if (candidate.equals("Candidate 3")) {
candidate3Votes++;
}
voteArea.setText(candidate + " received a vote from " + name + ".\n");
// Clear name field
nameField.setText("");
}
public static void main(String[] args) {
new VotingApp();
}
}
```
这个程序创建了一个投票应用程序,其中包含一个姓名文本框、一个候选人下拉框、一个投票按钮和一个显示投票结果的文本区域。当用户选择一个候选人并点击投票按钮时,该候选人的票数将增加,并且在文本区域中显示投票结果。
希望这能帮助到您!
阅读全文
相关推荐
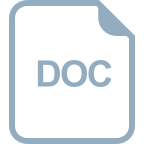
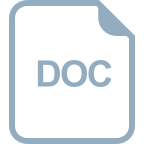
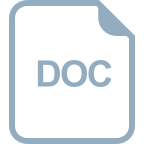
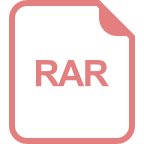
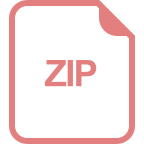
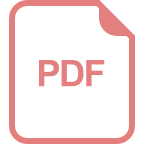
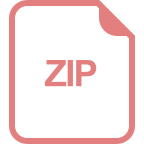
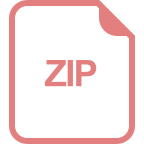
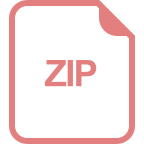
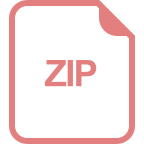
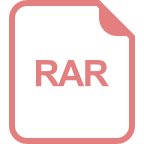
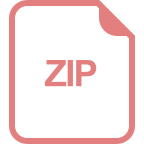
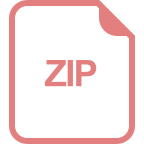
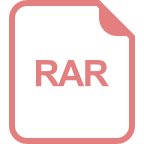
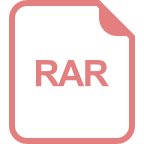
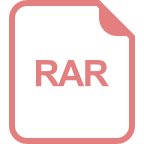
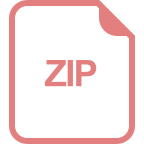
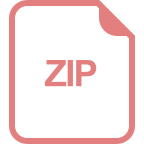
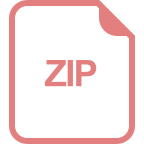