linux下用getopt_long实现ls命令的代码
时间: 2024-05-05 20:18:43 浏览: 94
可以参考以下代码:
```
#include <stdio.h>
#include <getopt.h>
#include <stdlib.h>
#include <unistd.h>
#include <dirent.h>
#include <sys/stat.h>
#include <string.h>
#define MAX_PATH 1024
static struct option long_options[] = {
{"all", no_argument, NULL, 'a'},
{"list", no_argument, NULL, 'l'},
{"help", no_argument, NULL, 'h'},
{NULL, 0, NULL, 0}
};
int main(int argc, char** argv) {
int opt;
int all_flag = 0, list_flag = 0;
char path[MAX_PATH] = ".";
while ((opt = getopt_long(argc, argv, "alh", long_options, NULL)) != -1) {
switch (opt) {
case 'a':
all_flag = 1;
break;
case 'l':
list_flag = 1;
break;
case 'h':
printf("Usage: ls [OPTION] [DIRECTORY]\n");
printf("List information about the FILEs (the current directory by default).\n\n");
printf("Options:\n");
printf(" -a, --all do not ignore entries starting with .\n");
printf(" -l, --list use a long listing format\n");
printf(" -h, --help display this help and exit\n");
exit(0);
default:
break;
}
}
if (argc > optind) {
strncpy(path, argv[optind], MAX_PATH);
}
DIR* dirp = opendir(path);
if (dirp == NULL) {
printf("Cannot open directory: %s\n", path);
exit(1);
}
struct dirent *dp;
while ((dp = readdir(dirp)) != NULL) {
if (all_flag == 0 && dp->d_name[0] == '.') {
continue;
}
char full_path[MAX_PATH];
snprintf(full_path, sizeof(full_path), "%s/%s", path, dp->d_name);
struct stat st;
if (lstat(full_path, &st) == -1) {
perror("lstat");
continue;
}
if (list_flag == 1) {
char buf[100];
strftime(buf, sizeof(buf), "%b %d %H:%M", localtime(&st.st_mtime));
printf("%c%s %ld %s %s %ld %s %s\n",
S_ISDIR(st.st_mode) ? 'd' : '-',
st.st_mode & S_IRUSR ? "r" : "-",
st.st_mode & S_IWUSR ? "w" : "-",
st.st_mode & S_IXUSR ? "x" : "-",
st.st_mode & S_IRGRP ? "r" : "-",
st.st_mode & S_IWGRP ? "w" : "-",
st.st_mode & S_IXGRP ? "x" : "-",
st.st_mode & S_IROTH ? "r" : "-",
st.st_mode & S_IWOTH ? "w" : "-",
st.st_mode & S_IXOTH ? "x" : "-",
st.st_nlink, getpwuid(st.st_uid)->pw_name,
getgrgid(st.st_gid)->gr_name,
st.st_size, buf, dp->d_name);
} else {
printf("%s ", dp->d_name);
}
}
closedir(dirp);
printf("\n");
return 0;
}
```
希望对你有所帮助!
阅读全文
相关推荐
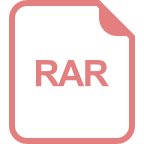
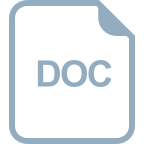
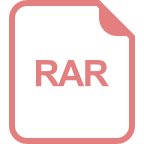
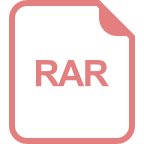
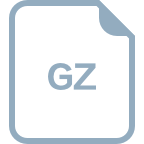
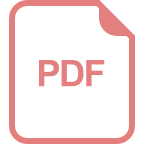
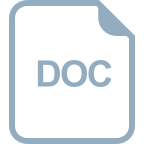
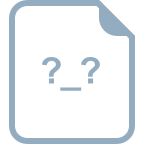
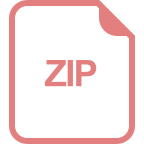
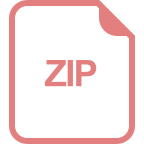
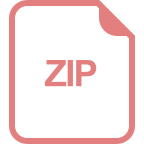