使用OpenCV-Python提取设定颜色、面积、圆度、惯性和凸度的特征点并圈出的代码示例
时间: 2023-07-04 18:24:09 浏览: 135
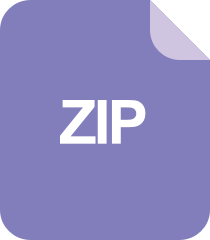
基于直方图特征提取和KNN的颜色分类器Python代码 使用OpenCV实现

以下是使用OpenCV-Python库提取设定颜色、面积、圆度、惯性和凸度的特征点并圈出的代码示例:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 将图像转换为HSV颜色空间
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
# 设定颜色范围
lower_color = np.array([0, 100, 100])
upper_color = np.array([10, 255, 255])
# 提取特定颜色区域
mask = cv2.inRange(hsv, lower_color, upper_color)
# 寻找特定颜色的特征点
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
# 计算特征点面积
area = cv2.contourArea(contour)
if area < 100 or area > 1000:
continue
# 计算特征点圆度
perimeter = cv2.arcLength(contour, True)
circularity = 4*np.pi*(area/(perimeter**2))
if circularity < 0.7:
continue
# 计算特征点惯性
M = cv2.moments(contour)
if M['m00'] == 0:
continue
cx = int(M['m10']/M['m00'])
cy = int(M['m01']/M['m00'])
inertia = cv2.moments(contour, True)
if inertia['nu20'] + inertia['nu02'] < 0.1:
continue
# 计算特征点凸度
hull = cv2.convexHull(contour)
hull_area = cv2.contourArea(hull)
if area/hull_area < 0.5:
continue
# 绘制特征点
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.circle(img, (cx, cy), 4, (0, 0, 255), -1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这些代码示例仅供参考,实际应用中需要根据具体的需求进行调整。
阅读全文
相关推荐
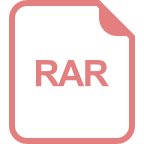
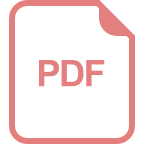
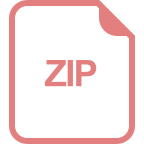
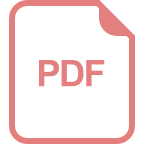
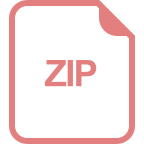
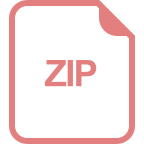
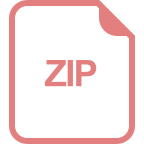
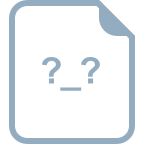
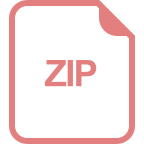
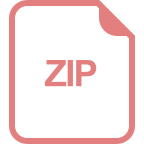
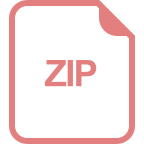
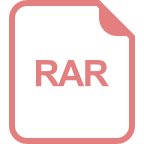
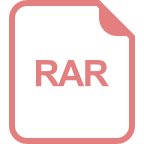
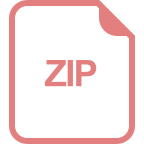
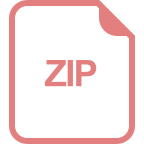
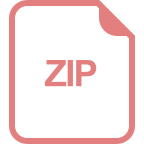