用Cpp写一个用户输入指定路径,程序写入一个名为hello.txt的记事本的程序
时间: 2023-09-05 11:04:41 浏览: 83
### 回答1:
好的,下面是使用 C++ 写一个程序,读入用户输入的路径,并在该路径下创建一个名为 hello.txt 的记事本的程序示例:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
// 声明文件路径变量
string filePath;
// 读入用户输入的文件路径
cout << "请输入文件路径:" << endl;
cin >> filePath;
// 在文件路径下创建名为 hello.txt 的文件
ofstream helloFile(filePath + "hello.txt");
// 向文件写入内容
helloFile << "Hello, World!" << endl;
// 关闭文件
helloFile.close();
return 0;
}
```
在上面的程序中,我们使用了 `ofstream` 类来创建并打开文件,并使用流插入运算符 (`<<`) 向文件写入内容。最后,我们使用 `close` 函数关闭文件。
希望这能帮到你。
### 回答2:
您可以使用以下代码来使用C++编写一个程序,该程序将用户输入的特定路径作为参数,并在该路径下创建一个名为hello.txt的记事本文件,并将其内容设置为"Hello, World!"。
```cpp
#include <iostream>
#include <fstream>
int main() {
// 提示用户输入指定路径
std::cout << "请输入路径:";
std::string path;
std::getline(std::cin, path);
// 创建文件路径
std::ofstream file(path + "\\hello.txt");
if (file.is_open()) {
// 写入文件内容
file << "Hello, World!";
file.close();
std::cout << "成功创建hello.txt文件!" << std::endl;
} else {
std::cout << "无法创建文件,请检查路径是否正确。" << std::endl;
}
return 0;
}
```
使用此程序,用户将被要求输入指定的路径,程序将根据提供的路径创建一个名为hello.txt的文件并写入"Hello, World!"。如果文件创建和写入过程成功,将显示一条成功的消息。否则,将显示一条错误消息。
请确保您在编译和运行该程序之前已经安装了适当的C++编译器,并且理解了如何在命令行或集成开发环境(IDE)中运行C++程序。
### 回答3:
使用Cpp编写一个程序,根据用户输入的路径,将内容写入一个名为hello.txt的记事本文件。
首先,我们需要包含头文件`<iostream>`和`<fstream>`,分别用于输入输出和文件操作。
然后,声明一个`main()`函数作为程序的入口函数。
在`main()`函数中,首先声明一个`string`类型的变量`path`用于接收用户输入的路径。
使用`cout`输出提示用户输入路径的信息,然后使用`cin`接收用户的输入,并将其赋值给`path`变量。
接下来,使用`ofstream`类的对象`outfile`来打开一个名为`hello.txt`的文件,以便于写入内容。打开文件的方式为输出模式,即`ofstream`类的构造函数传入参数`ios::out`。
如果文件打开成功,则使用`outfile`对象的`<<`操作符将用户输入的内容写入文件中。写入的内容可以是任意格式和长度,例如 "Hello, World!"。
写入完成后,使用`outfile.close()`关闭文件。
最后,返回0表示程序成功运行结束。
下面是完整的代码:
```cpp
#include <iostream>
#include <fstream>
int main() {
std::string path;
std::cout << "请输入路径:";
std::cin >> path;
std::ofstream outfile(path + "\\hello.txt", std::ios::out);
if (outfile.is_open()) {
outfile << "Hello, World!";
outfile.close();
}
return 0;
}
```
请注意,以上代码只是一个简单的示例,仅供参考。实际使用时,我们需要进行错误检查,以确保文件能够成功打开和写入。
阅读全文
相关推荐
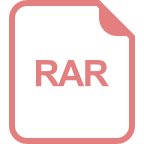
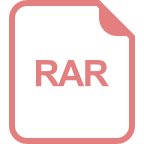
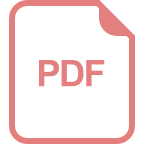
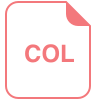
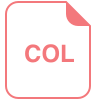




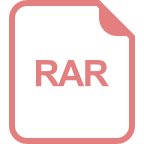
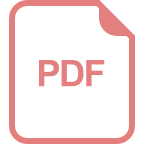