c语言水果商店库存管理系统代码
时间: 2023-11-03 14:58:00 浏览: 86
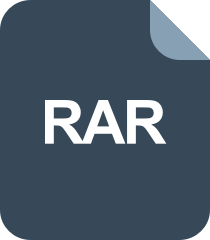
水果库存管理系统
以下是一个简单的C语言水果商店库存管理系统代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FRUITS 100
typedef struct {
char name[20];
int quantity;
float price;
} Fruit;
Fruit fruits[MAX_FRUITS];
int num_fruits = 0;
void add_fruit() {
if (num_fruits == MAX_FRUITS) {
printf("Error: Maximum number of fruits reached.\n");
return;
}
Fruit new_fruit;
printf("Enter the name of the fruit: ");
scanf("%s", new_fruit.name);
printf("Enter the quantity of the fruit: ");
scanf("%d", &new_fruit.quantity);
printf("Enter the price of the fruit: ");
scanf("%f", &new_fruit.price);
fruits[num_fruits] = new_fruit;
num_fruits++;
printf("Fruit added successfully.\n");
}
void remove_fruit() {
char name[20];
printf("Enter the name of the fruit to remove: ");
scanf("%s", name);
int found = 0;
for (int i = 0; i < num_fruits; i++) {
if (strcmp(name, fruits[i].name) == 0) {
found = 1;
for (int j = i; j < num_fruits - 1; j++) {
fruits[j] = fruits[j + 1];
}
num_fruits--;
printf("Fruit removed successfully.\n");
break;
}
}
if (!found) {
printf("Error: Fruit not found.\n");
}
}
void display_fruits() {
printf("Name\tQuantity\tPrice\n");
for (int i = 0; i < num_fruits; i++) {
printf("%s\t%d\t\t%.2f\n", fruits[i].name, fruits[i].quantity, fruits[i].price);
}
}
int main() {
int option = 0;
while (option != 4) {
printf("1. Add a fruit\n");
printf("2. Remove a fruit\n");
printf("3. Display all fruits\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &option);
switch (option) {
case 1:
add_fruit();
break;
case 2:
remove_fruit();
break;
case 3:
display_fruits();
break;
case 4:
printf("Exiting...\n");
break;
default:
printf("Invalid option.\n");
break;
}
}
return 0;
}
```
此代码允许用户添加、删除和显示水果库存。它使用结构体来存储每个水果的名称、数量和价格。用户可以通过菜单选择操作,并可以在需要时退出程序。
阅读全文
相关推荐
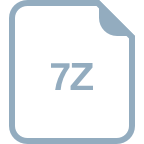

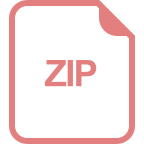

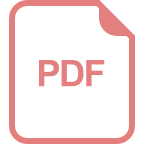
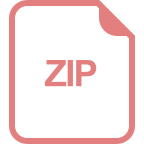
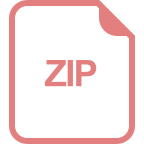
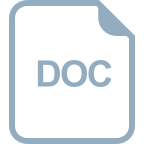
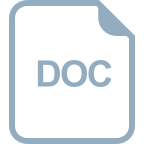

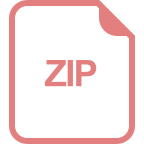
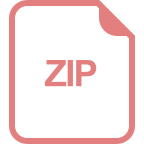