the socket name is not available on this system
时间: 2024-05-20 20:16:38 浏览: 10
As an AI language model, I don't have enough context to provide a specific answer. However, "the socket name is not available on this system" typically indicates that a network socket, which is an endpoint for sending or receiving data across a computer network, cannot be created due to a conflict with another application or service already using the same port number. This error message can also occur if the socket name is misspelled or if the required socket library is not installed on the system. To resolve this issue, you may need to change the port number or check if any conflicting applications are running.
相关问题
NameError: name 'socket' is not defined
NameError: name 'socket' is not defined 错误通常是由于在代码中使用了未定义的变量或模块。这可能是由于拼写错误、未导入模块或未正确安装模块等原因引起的。
解决此错误的方法包括:
1.检查代码中是否存在拼写错误或语法错误。
2.确保使用的模块已正确导入,并且已正确安装。
3.尝试重新安装相关模块,以确保其正确安装。
this.socket.close is not a function
This error message suggests that the method `close()` is not available on the `socket` object in your code.
There are a few reasons why this could be happening:
1. The `socket` object is not defined: Make sure that you have defined the `socket` object before calling the `close()` method.
2. The `socket` object is not an instance of a class that has a `close()` method: Check the documentation of the library or framework you are using to see if the `socket` object has a `close()` method. If not, you may need to use a different method to close the connection.
3. The `socket` object has already been closed: If you have already closed the socket connection, then calling `close()` again will result in an error. Make sure to check if the socket is open before attempting to close it again.
Without seeing your code, it is difficult to provide a more specific solution.
相关推荐
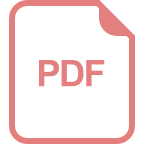
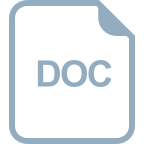
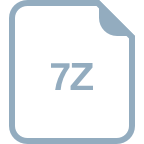












