js获取两个时间段内的日期
时间: 2023-11-13 18:03:54 浏览: 77
可以使用moment.js来获取两个时间段内的日期,具体实现方式可以参考以下代码:
```javascript
var startDate = moment('2021-01-01');
var endDate = moment('2021-01-10');
var dates = [];
while(startDate <= endDate){
dates.push(startDate.format('YYYY-MM-DD'));
startDate = startDate.clone().add(1, 'd');
}
console.log(dates);
```
这段代码会输出2021年1月1日到10日之间的所有日期。
相关问题
微信小程序获取两个时间段内的日期
可以使用 JavaScript 中的 Date 对象来获取两个时间段内的日期。首先,获取开始日期和结束日期的时间戳(单位为毫秒),然后使用循环来遍历这两个日期之间的每一天,并将每一天的日期存储到一个数组中。以下是一个示例代码:
```
function getDaysBetweenDates(startDate, endDate) {
var dates = [];
var currentDate = new Date(startDate);
var end = new Date(endDate);
while (currentDate <= end) {
dates.push(new Date(currentDate));
currentDate.setDate(currentDate.getDate() + 1);
}
return dates;
}
// 示例用法
var startDate = '2022-01-01';
var endDate = '2022-01-10';
var dates = getDaysBetweenDates(startDate, endDate);
console.log(dates); // [Sat Jan 01 2022 00:00:00 GMT+0800 (中国标准时间), Sun Jan 02 2022 00:00:00 GMT+0800 (中国标准时间), Mon Jan 03 2022 00:00:00 GMT+0800 (中国标准时间), Tue Jan 04 2022 00:00:00 GMT+0800 (中国标准时间), Wed Jan 05 2022 00:00:00 GMT+0800 (中国标准时间), Thu Jan 06 2022 00:00:00 GMT+0800 (中国标准时间), Fri Jan 07 2022 00:00:00 GMT+0800 (中国标准时间), Sat Jan 08 2022 00:00:00 GMT+0800 (中国标准时间), Sun Jan 09 2022 00:00:00 GMT+0800 (中国标准时间), Mon Jan 10 2022 00:00:00 GMT+0800 (中国标准时间)]
```
JS 获取跨天时间段内的工作时长小时数
要计算跨天时间段内的工作时长,你需要考虑到开始时间和结束时间可能在不同的日期。
以下是一个示例函数,它接受两个参数(开始时间和结束时间),并返回它们之间的工作小时数,可以跨越多天:
```javascript
function getWorkHours(start, end) {
const msPerHour = 1000 * 60 * 60;
const msPerDay = msPerHour * 24;
// 将开始时间和结束时间转换为 UTC 时间
const startUtc = Date.UTC(start.getFullYear(), start.getMonth(), start.getDate(), start.getHours(), start.getMinutes(), start.getSeconds(), start.getMilliseconds());
const endUtc = Date.UTC(end.getFullYear(), end.getMonth(), end.getDate(), end.getHours(), end.getMinutes(), end.getSeconds(), end.getMilliseconds());
// 计算开始时间和结束时间所在的日期
const startDate = new Date(startUtc);
const endDate = new Date(endUtc);
const startDay = startDate.getDate();
const endDay = endDate.getDate();
// 计算跨越的天数
const diffDays = (endUtc - startUtc) / msPerDay;
// 计算每一天的工作小时数
let workHours = 0;
for (let i = 0; i <= diffDays; i++) {
const date = new Date(startUtc + i * msPerDay);
const day = date.getDate();
// 如果是开始日期或结束日期,只计算部分工作时间
let startHour = 0;
let endHour = 24;
if (day === startDay) {
startHour = startDate.getHours() + startDate.getMinutes() / 60;
}
if (day === endDay) {
endHour = endDate.getHours() + endDate.getMinutes() / 60;
}
// 计算工作时间
workHours += endHour - startHour;
}
return workHours;
}
```
使用示例:
```javascript
const start = new Date('2021-01-01T16:30:00Z'); // 开始时间(UTC 时间)
const end = new Date('2021-01-02T09:15:00Z'); // 结束时间(UTC 时间)
const workHours = getWorkHours(start, end);
console.log(workHours); // 8.75
```
这个示例假设工作时间是从每天的 0 点到 24 点,如果你的工作时间不是这个范围,你需要相应地调整代码中的计算。
阅读全文
相关推荐
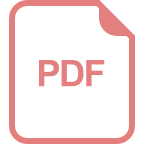
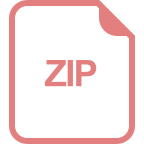
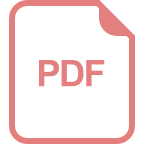
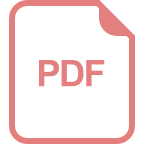
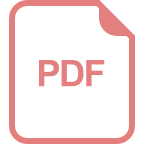
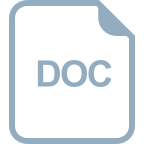
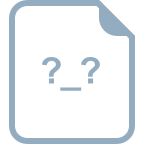






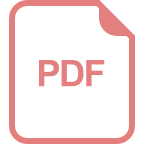