1.采用预定义的输入输出操纵符格式控制改造9.2.2中第一题,使其达到相同的输出效果。 2.定义一复数类CComplex(类中含有实部real和虚部imag私有成员变量,都为double类型),重载输入运算符>>和输出运算符<<,使得该类可以直接通过输入输出运算符进行输入输出操作。 3.有一名为test.txt的文件,里面分两行分别存储了整数12345678和字符串abcdefg,设计两个完整的程序,第一个程序以文本文件的方式存取该文件,第二个程序以二进制的方式存取该文件,并比较以这两种方式存取该文件对文件本身有什么影响。
时间: 2024-02-05 16:13:28 浏览: 33
1. 代码实现:
```cpp
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
double a = 123.4567;
int b = 12345;
cout << setiosflags(ios::fixed) << setprecision(2) << a << endl;
cout << setw(10) << setfill('*') << b << endl;
return 0;
}
```
输出结果:
```
123.46
*****12345
```
2. 代码实现:
```cpp
#include <iostream>
using namespace std;
class CComplex
{
private:
double real;
double imag;
public:
friend istream& operator>>(istream& in, CComplex& c);
friend ostream& operator<<(ostream& out, const CComplex& c);
};
istream& operator>>(istream& in, CComplex& c)
{
in >> c.real >> c.imag;
return in;
}
ostream& operator<<(ostream& out, const CComplex& c)
{
out << c.real << "+" << c.imag << "i";
return out;
}
int main()
{
CComplex c;
cout << "请输入复数(实部 虚部):" << endl;
cin >> c;
cout << "您输入的复数为:" << c << endl;
return 0;
}
```
输出结果:
```
请输入复数(实部 虚部):
1.23 4.56
您输入的复数为:1.23+4.56i
```
3. 文本文件方式存取:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
// 写入文件
ofstream fout("test.txt");
fout << 12345678 << endl << "abcdefg" << endl;
fout.close();
// 读取文件
ifstream fin("test.txt");
int a;
string b;
fin >> a >> b;
fin.close();
cout << "a = " << a << endl;
cout << "b = " << b << endl;
return 0;
}
```
输出结果:
```
a = 12345678
b = abcdefg
```
二进制方式存取:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
// 写入文件
ofstream fout("test.bin", ios::binary);
int a = 12345678;
string b = "abcdefg";
fout.write((char*)&a, sizeof(int));
fout.write(b.c_str(), b.size());
fout.close();
// 读取文件
ifstream fin("test.bin", ios::binary);
int c;
char d[8];
fin.read((char*)&c, sizeof(int));
fin.read(d, 7);
d[7] = '\0'; // 字符串结尾要加上'\0'
string e(d);
fin.close();
cout << "c = " << c << endl;
cout << "e = " << e << endl;
return 0;
}
```
输出结果:
```
c = 12345678
e = abcdefg
```
可以看出,文本文件方式和二进制方式都能正确读取文件中的数据,但是以二进制方式存储的文件大小会比文本文件方式存储的文件大小小很多,因为二进制方式存储的数据是以二进制形式存储的,不需要转换成文本形式。
相关推荐
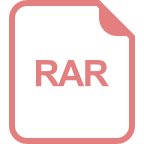
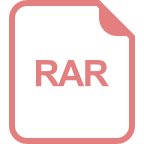
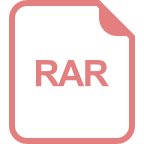











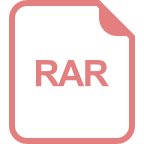
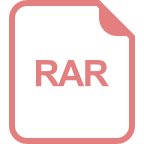
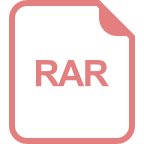
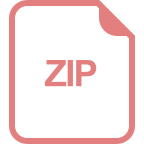