0/1背包问题为什么不能用贪心算法求解
时间: 2023-11-06 19:46:59 浏览: 105
0/1背包问题是一个经典的动态规划问题,使用贪心算法无法得到最优解。
贪心算法的思想是每次选择当前最优解,但是在0/1背包问题中,如果仅仅选择当前最大的物品,可能会导致后续无法放下更多的物品,从而得到不是最优解的结果。
举个例子,假设背包容量为10,现在有三个物品,重量分别为6、4、3,价值分别为8、7、5。如果使用贪心算法,我们会优先选择重量为6的物品,但是这样就无法放下其他物品了,得到的最优解为8。然而,如果我们先选择重量为4的物品,再选择重量为3的物品,就可以将背包放满,得到的最优解为12。
因此,0/1背包问题需要使用动态规划等复杂算法来求解。
相关问题
用C语言实现贪心算法求解简单0/1背包问题
好的,我来为您介绍一下如何使用C语言实现贪心算法来求解简单的0/1背包问题。
贪心算法是一种在每一步选择中都采取在当前状态下最好或最优的选择,从而希望导致结果是全局最好或最优的算法。对于0/1背包问题,贪心算法并不能保证得到最优解,但在某些情况下可以给出一个近似解。
以下是使用C语言实现贪心算法求解0/1背包问题的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义物品结构体
typedef struct {
int value;
int weight;
float ratio;
} Item;
// 比较函数,用于按价值密度降序排序
int compare(const void *a, const void *b) {
Item *itemA = (Item *)a;
Item *itemB = (Item *)b;
if (itemB->ratio > itemA->ratio) return 1;
else if (itemB->ratio < itemA->ratio) return -1;
else return 0;
}
// 贪心算法求解0/1背包问题
float knapsack_greedy(int n, int capacity, Item items[]) {
// 按价值密度降序排序
qsort(items, n, sizeof(Item), compare);
int curWeight = 0;
float totalValue = 0.0;
for (int i = 0; i < n; i++) {
if (curWeight + items[i].weight <= capacity) {
curWeight += items[i].weight;
totalValue += items[i].value;
} else {
// 装入部分物品
int remaining = capacity - curWeight;
totalValue += items[i].value * ((float)remaining / items[i].weight);
break;
}
}
return totalValue;
}
int main() {
int n, capacity;
printf("请输入物品数量和背包容量: ");
scanf("%d %d", &n, &capacity);
Item items[n];
printf("请输入每个物品的价值和重量:\n");
for (int i = 0; i < n; i++) {
scanf("%d %d", &items[i].value, &items[i].weight);
items[i].ratio = (float)items[i].value / items[i].weight;
}
float maxValue = knapsack_greedy(n, capacity, items);
printf("贪心算法求解的最大价值: %.2f\n", maxValue);
return 0;
}
```
这段代码的主要步骤如下:
1. 定义物品结构体,包含价值、重量和价值密度。
2. 编写比较函数,用于按价值密度降序排序。
3. 实现贪心算法函数:
- 首先按价值密度降序排序所有物品。
- 然后依次选择物品,直到装满背包或所有物品都被考虑。
- 如果无法装入整个物品,则装入部分物品。
4. 在main函数中,读取输入数据,调用贪心算法函数,并输出结果。
需要注意的是,贪心算法并不总是能得到0/1背包问题的最优解。对于某些特殊情况,如所有物品的价值密度相同,贪心算法可能会得到次优解。因此,在实际应用中,应该根据具体情况选择合适的算法。
背包问题为什么不能使用贪心算法
背包问题不能使用贪心算法的原因是贪心算法在某些情况下无法得到最优解。在0-1背包问题中,物品是不可分割的,意味着背包不一定能装满。贪心算法通常会选择当前最优的物品放入背包,但这种策略可能导致背包容量没有被充分利用,从而无法得到最优解。
在0-1背包问题中,我们需要在给定的背包容量下选择一些物品放入背包,使得物品的总价值最大化。由于物品是不可分割的,我们只能选择将物品完整地放入背包或者不放入背包。这就导致了贪心算法的局限性,因为贪心算法只考虑当前最优的选择,而没有考虑到后续的选择可能会导致更优的结果。
为了解决0-1背包问题,我们可以考虑使用枚举、回溯、动态规划等方法来求解。这些方法可以考虑所有可能的选择,并通过比较得出最优解。这样可以确保我们找到的解是最优的。
阅读全文
相关推荐



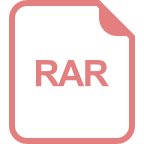












