c# winform数码管控件
时间: 2023-08-16 13:04:19 浏览: 72
C# WinForm中没有内置的数码管控件,但您可以使用自定义控件来实现数码管效果。以下是一个简单的示例:
1. 创建一个新的 WinForm 应用程序项目。
2. 右键单击项目,选择“添加”>“用户控件”。
3. 将控件重命名为“SevenSegmentDisplay”。
4. 在 SevenSegmentDisplay.cs 文件中,添加以下代码:
```
using System.Drawing;
using System.Windows.Forms;
public partial class SevenSegmentDisplay : UserControl
{
private bool[] segments = new bool[7];
public bool[] Segments
{
get { return segments; }
set { segments = value; }
}
public SevenSegmentDisplay()
{
InitializeComponent();
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Graphics g = e.Graphics;
g.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
// Draw seven segments
int segmentWidth = this.Width / 4;
int segmentHeight = this.Height / 7;
int x = 0;
int y = 0;
// Segment A
if (segments[0])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x, y), new Point(x + segmentWidth, y), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight / 2), new Point(x + segmentHeight / 2, y + segmentHeight / 2) });
x += segmentWidth;
// Segment B
if (segments[1])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x + segmentHeight / 2, y), new Point(x + segmentWidth - segmentHeight / 2, y), new Point(x + segmentWidth, y + segmentHeight / 2), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight), new Point(x + segmentHeight / 2, y + segmentHeight) });
x += segmentWidth;
// Segment C
if (segments[2])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x + segmentHeight / 2, y), new Point(x + segmentWidth - segmentHeight / 2, y), new Point(x + segmentWidth, y + segmentHeight / 2), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight), new Point(x + segmentHeight / 2, y + segmentHeight) });
x += segmentWidth;
// Segment D
if (segments[3])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x, y), new Point(x + segmentWidth, y), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight / 2), new Point(x + segmentHeight / 2, y + segmentHeight / 2) });
x = 0;
y += segmentHeight;
// Segment E
if (segments[4])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x + segmentHeight / 2, y), new Point(x + segmentWidth - segmentHeight / 2, y), new Point(x + segmentWidth, y + segmentHeight / 2), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight), new Point(x + segmentHeight / 2, y + segmentHeight) });
x += segmentWidth;
// Segment F
if (segments[5])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x + segmentHeight / 2, y), new Point(x + segmentWidth - segmentHeight / 2, y), new Point(x + segmentWidth, y + segmentHeight / 2), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight), new Point(x + segmentHeight / 2, y + segmentHeight) });
x += segmentWidth;
// Segment G
if (segments[6])
g.FillPolygon(Brushes.Red, new Point[] { new Point(x, y + segmentHeight / 2), new Point(x + segmentWidth - segmentHeight / 2, y + segmentHeight / 2), new Point(x + segmentWidth, y + segmentHeight / 2 + segmentHeight / 2), new Point(x + segmentWidth / 2, y + segmentHeight), new Point(x + segmentHeight / 2, y + segmentHeight / 2 + segmentHeight / 2) });
}
}
```
5. 在 SevenSegmentDisplay.Designer.cs 文件中,添加以下代码:
```
partial class SevenSegmentDisplay
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.SuspendLayout();
//
// SevenSegmentDisplay
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.BackColor = System.Drawing.Color.Black;
this.Name = "SevenSegmentDisplay";
this.Size = new System.Drawing.Size(150, 250);
this.ResumeLayout(false);
}
#endregion
}
```
6. 在您的主窗体上,添加一个 SevenSegmentDisplay 控件。
7. 在您的代码中,设置 SevenSegmentDisplay 控件的 Segments 属性,以显示所需的数字。
例如,要显示数字“8”,可以使用以下代码:
```
SevenSegmentDisplay1.Segments = new bool[] { true, true, true, true, true, true, true };
```
这将在 SevenSegmentDisplay 控件上显示一个数字“8”。
相关推荐
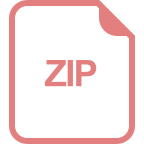














