请用C语言编程实现对带头结点的单链表进行基本操作的系统,主要包括表的创建,输入,查询,取值。插入,删除和输出等操作
时间: 2024-05-21 21:10:54 浏览: 35
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
}Node, *LinkedList;
LinkedList createList(); // 创建链表
void inputList(LinkedList list); // 输入链表
void printList(LinkedList list); // 输出链表
Node *searchList(LinkedList list, int target); // 查询链表
int getValue(LinkedList list, int pos); // 取值
void insertList(LinkedList list, int pos, int value); // 插入链表
void deleteList(LinkedList list, int pos); // 删除链表
int main() {
LinkedList list = createList();
inputList(list);
printf("链表内容:\n");
printList(list);
int target;
printf("请输入要查询的值:");
scanf("%d", &target);
Node *node = searchList(list, target);
if (node != NULL) {
printf("找到了,值为:%d\n", node->data);
} else {
printf("未找到\n");
}
int pos;
printf("请输入要取值的位置:");
scanf("%d", &pos);
int value = getValue(list, pos);
printf("取得的值为:%d\n", value);
int insertPos, insertValue;
printf("请输入要插入的位置和值:");
scanf("%d%d", &insertPos, &insertValue);
insertList(list, insertPos, insertValue);
printf("插入后的链表内容:\n");
printList(list);
int deletePos;
printf("请输入要删除的位置:");
scanf("%d", &deletePos);
deleteList(list, deletePos);
printf("删除后的链表内容:\n");
printList(list);
return 0;
}
// 创建链表
LinkedList createList() {
LinkedList head = (LinkedList)malloc(sizeof(Node));
head->next = NULL;
return head;
}
// 输入链表
void inputList(LinkedList list) {
printf("请输入链表的长度:");
int length;
scanf("%d", &length);
LinkedList p = list;
for (int i = 0; i < length; i++) {
printf("请输入第%d个节点的值:", i + 1);
int value;
scanf("%d", &value);
LinkedList node = (LinkedList)malloc(sizeof(Node));
node->data = value;
node->next = NULL;
p->next = node;
p = node;
}
}
// 输出链表
void printList(LinkedList list) {
LinkedList p = list->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 查询链表
Node *searchList(LinkedList list, int target) {
LinkedList p = list->next;
while (p != NULL) {
if (p->data == target) {
return p;
}
p = p->next;
}
return NULL;
}
// 取值
int getValue(LinkedList list, int pos) {
LinkedList p = list->next;
int i = 1;
while (p != NULL && i < pos) {
p = p->next;
i++;
}
if (p == NULL || i > pos) {
printf("取值位置无效\n");
return -1;
} else {
return p->data;
}
}
// 插入链表
void insertList(LinkedList list, int pos, int value) {
LinkedList p = list;
int i = 0;
while (p != NULL && i < pos - 1) {
p = p->next;
i++;
}
if (p == NULL || i > pos - 1) {
printf("插入位置无效\n");
} else {
LinkedList node = (LinkedList)malloc(sizeof(Node));
node->data = value;
node->next = p->next;
p->next = node;
}
}
// 删除链表
void deleteList(LinkedList list, int pos) {
LinkedList p = list;
int i = 0;
while (p != NULL && i < pos - 1) {
p = p->next;
i++;
}
if (p == NULL || i > pos - 1 || p->next == NULL) {
printf("删除位置无效\n");
} else {
LinkedList q = p->next;
p->next = q->next;
free(q);
}
}
相关推荐
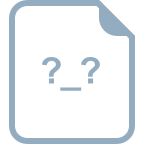














